One of the core things I've been working on for the past 10 years are APIs. Everything from simple APIs that are used by one client to multi device and multi purpose APIs. During those years I've also had the opportunity to work with many third party APIs like Stripe, Twilio and others who are less popular and glamorous ๐. Almost all of those APIs were REST based and unique in some way.
There are many reasons why REST is so popular. It's simple to understand, it's flexible, it works on any scale, it has a great community and tools built around it. But besides those I'd also say that a lot of popularity came from the fact that's it's oldest rival, SOAP, is just horrible. If you've ever used or worked with SOAP - you know! The clients were terrible, people did what they wanted with XML, it's bulky, the auth situation was sometimes wacky...Thankfully JSON and REST based APIs won that battle.
On the other hand there are also newer, more modern players that are trying to take some of that spotlight from REST based APIs. I'm of course talking about GraphQL. GraphQL is also JSON based and shares some of the good traits that REST has like flexibility, performance, scalability...For me personally the two key downsides of GraphQL are that it's built by Facebook and that it shifts the API design process to the client. What I mean by that is that it leaves mobile devs and front end devs in charge of building their own ad hoc APIs and making queries on the database. Don't know about you but I don't think that's the best idea. Don't get me wrong, I love mobile and front end devs, but they might not have a lot of experience with database design, writing queries and building APIs. You probably wouldn't give your back-end dev to design an app, a car mechanic to fix an airplane or a veterinarian to operate on a human person. Could they do it - maybe, possibly. Should they - I don't think so.
In my opinion REST is still king of the jungle and it's not going to be dethroned any time soon. The only problem with REST is that at the end of the day it's not a standard nor a protocol. Rather, it's a set of "architectural constraints". What a fancy way of not calling something a standard ๐คฃ But that right there tickles the imagination of many. They tend to do their own thing or implement those constraints based on their own (miss)understanding. To avoid such misunderstandings I've decided to write down what I think are the Ten REST API Commandments. Follow these and you'll enjoy love from your mobile devs, admiration from other fellow back-end devs and a rockstar status on Twitter ๐.
So like the song says: Won't you follow me into the jungle?
1. Be Practical
It's really simple. If you're building a REST API you should accept and respond with JSON. Not XML. Not something else. JSON.
Yes, JSON is not "required" because REST isn't a standard but it's one of those things you just do. Just like you put on clothes everyday, go to sleep each night or drink a shot of rakija everyday. Well maybe not the last part but you get the point ๐ The sooner we get everybody off of XML and on to JSON the better it will be for everyone. In case you're not sure why JSON is better, let me just casually name a few reasons:
- it's easier to use, write and read - literary anyone and you grandma can read it
- it's faster and consumes less memory space
- it doesn't need special dependencies or packages to parse it
- every single meaningful programming language has great support for it
If this still seems abstract I invite you to download a random XML file from the internet and try to parse it. As someone who had to do it way too many times in the 2000s, I can assure you, you will come back crying to JSON. There simply is no reason why you'd use XML instead of JSON in 2021...Yes, if you have a legacy enterprise system then I feel your pain, but even that is changing. More and more of these big and outdated enterprises are updating their internal and external APIs from XML and SOAP to JSON and REST.
JSON shouldn't just be used on the response side of things but also on the request side. So instead of sending the data using form-data or x-www-form-urlencoded send it as JSON. It's just simpler to read, write, test and manage for everybody.
Remember - when in doubt - use JSON. Thank you from all us developers in advance.
2. Be Methodical
You wouldn't believe how many times I saw APIs that used only GET methods for everything from storing data to filtering ๐คช As a developer you should always strive to understand the tools you are using so it's important to know how exactly HTTP works if you are developing APIs. Each HTTP method has been designed to be used in certain situations. Let's go through each of them and see when you should use each of them.
Before we start just a small but fun clarification. When describing HTTP methods people often use words like "safe" and "idempotent". These sound really nice and mystical but it's not that complicated. In plain English, safe means ready only. You can make requests to that endpoint without having to worry about updating, destroying or changing values. Idempotent on the other hand, means that you can make multiple requests to the same endpoint without changing something or getting different results. Generally speaking all safe methods are also idempotent at the same time but not all idempotent methods are safe ๐. I know it's confusing at first but we'll explain below.
GET
You should use the GET method when you wish to read data. Not store, not update, not change data. Only read the data. The concept is super simple and shouldn't confuse anyone. Now that we know that GET methods should be used ONLY for reading data and that they always return the same data we can say that GET requests are safe and idempotent.
POST
When you make a POST request everyone in the world expects you to usually STORE something. This means you will create a new row in the database, write something somewhere or create something from nothing if you will. You can send data to a POST method using multiple content types: multipart/form-data or x-www-form-urlencoded or raw (application/json, text/plain...etc). When building REST APIs I'd suggest the clients send data as application/json. This way we are keeping it consistent and in the JSON spirit but also sending JSON data allows you to make really complicated requests with ease. Finally POST operations are not safe because they do change things on the server side and they are certainly not idempotent because making two requests to the same endpoint will result in different resources.
PUT
PUT requests are most often used in the context of updating things. It could also be used to create new records but then the idea would be that the client should be the one defining the ID of the new resource. So to make your life easier simply use PUT when you wish to update a resource. PUT is of course not a safe operation because it makes changes on the server side but, and you'll love this, it's an idempotent operation.
DELETE
This one is self explanatory I'd say. When you wish to delete resources simply use the DELETE method. It's of course not a safe operation but some say it's idempotent and some say it's not.
PATCH
A patch request is meant to be used to update resources again but unlike PUT it should update only the changed data whereas PUT can and should update the entire resource. It's not safe nor is it idempotent.
Now that we know the basics, here is what happens in the real world. Most people use GET, POST and some use PUT and DELETE. Rarely do I see PATCH being used. I'd advise you to use all of the HTTP methods available to you because that is why they were designed for. You can literally map any CRUD operation to POST, GET, UPDATE and DELETE. I simply beg of you don't ever use GET to create or update data. Don't.
3. Be Semantical
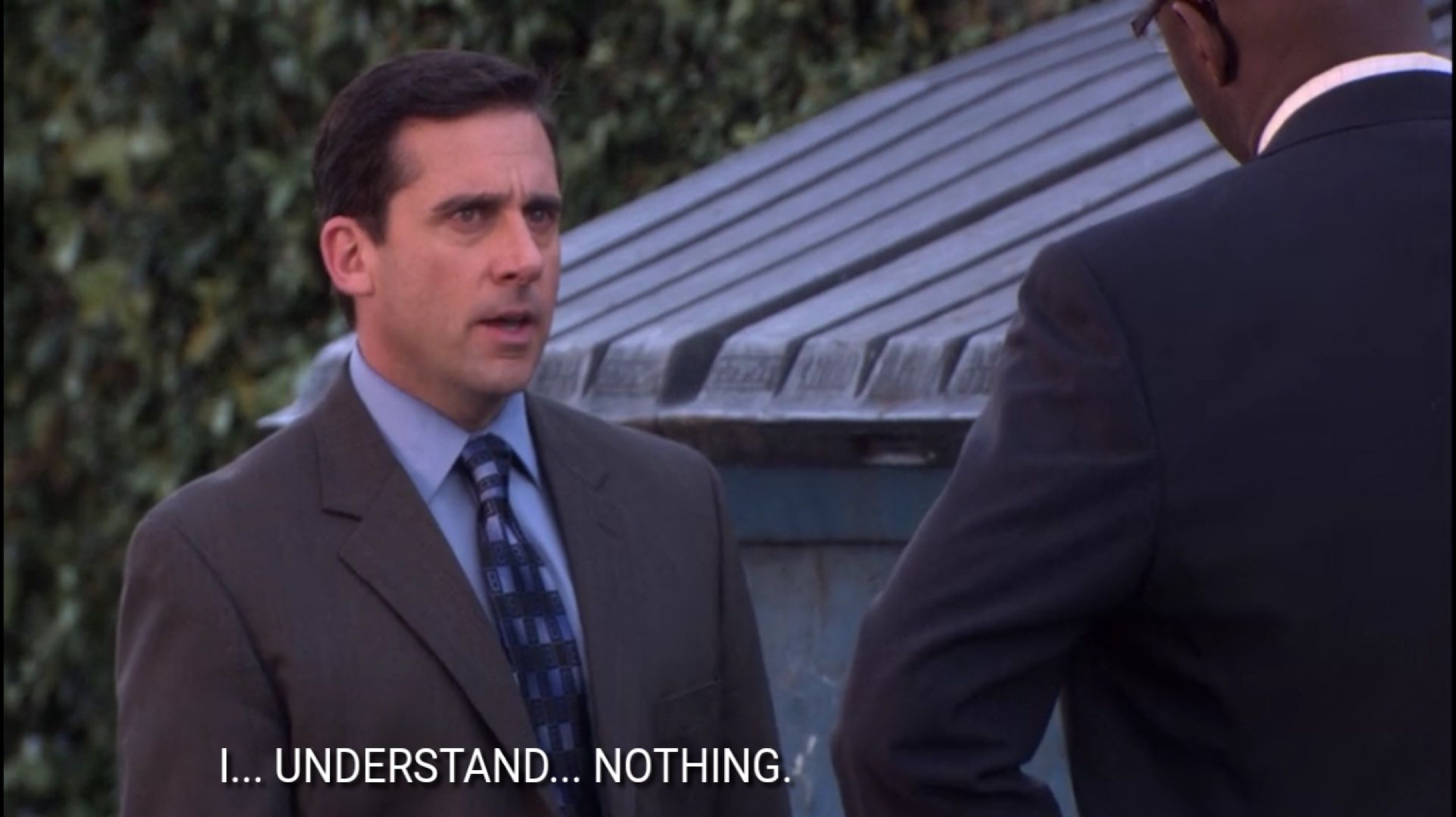
This is probably the only time you'll hear me recommending someone to be semantical. But in this case it's important and it's all about naming things correctly. Oftentimes I've seen API documentation with horrible naming conventions for everything. In my opinion every great REST APi should be easily understandable by your average Joe. Starting from endpoint names to input parameters all the way to the JSON keys.
So let's start with API endpoints. The rules here are also quite simple:
- use nouns instead of verbs
- use plural instead of singular
The reason why you should use nouns is because when you are making an HTTP request, as we saw above, you are already using verbs. Each HTTP method (GET, POST, PUT, PATCH) is a verb in the English language. Hence it makes no sense to have double verbs - doesn't it!? If you named an API endpoint as /getUsers
and you were making a GET request to view all the users when you read that, out loud, it sounds funny. GET /getUsers
. Makes no sense.
Another common theme I kept seeing is the use of singular instead of plural for the endpoint names. That of course couldn't be more wrong. You want your API endpoints to be consistent, simple and logical. If you use plural then for every HTTP method you can use the same URI. If you do GET /users
you are listing all the users and if you do POST /users
you are creating a user. So again, same URI, different HTTP methods (verbs). It gets even cooler if you wish to view the details of a single user - you request GET /users/:id
to fetch the information. So as you can see - it's still the same starting resource name, it just has more depth. If you used singular then GET /user
would mean that you wish to get a single user and you would need more different URIs for other cases. Plural just makes way more sense.
To recap, let's take a look at a couple of good and bad examples just so we are 100% clear.
Good ๐๐ป
GET /users
POST /users
GET /users/23
PUT /users/23
DELETE /users/23
GET /users/23/comments
Bad ๐๐ป
GET /user/23
GET /listAllUsers
POST /user/create
PUT /updateUser/23
GET /userComments/23
I think you GET the idea ๐. It's really simple and just by looking and reading the examples above you know which ones sound good and make more sense.
A few bonus notes regarding endpoint naming: try to use single words instead of multi words. If you really have to use multi words then use hyphens between them. And for the love of the god use all lowercase letters in URIs.
To wrap up this section, I'd like to quickly go over the naming conventions for JSON keys in the request and response data. There is much debate around this, especially given that there are 3 possible scenarios: camelCase, snake_case and spinal-case. Again nobody can stop you from using any of these, theoretically you wouldn't be wrong. But, I would recommend using snake_case. Why? Stripe uses it. PayPal uses it. Facebook uses it...You get the point. Personally, I find snake_case much easier to read and that is why all of our APIs use snake_case. There have also been studies like this one which gave an advantage to snake_case over camelCase in terms of readability.
4. Be Secure
I will just come out and say it: if you are not using HTTPs in 2021. then shame on you. Your REST API should be running on HTTPs without any questions. Using HTTPs simply provides an element of security that you don't get with HTTP. It saves your users from man-in-the-middle-attacks and encrypts communication between the client and the API. Many other big players like Apple and Google will soon force you to have HTTPs enabled. The best part of it is that nowadays you can get an SSL certificate for free. Using services like AWS and Route 53, the Azure equivalent or a service called Let's encrypt. They all work great, I can personally attest to that. You can always also buy a premium SSL certificate. Whatever you do, just use HTTPs on your next API. You'll thank me later.
Another thing that saddens me the most is when I see an API isn't using any form of authorization. If you are building an API you simply need to be able to control who has access to it. It's so simple but many people don't do it because they feel like it's too complicated to implement, use and maintain. It really doesn't have to be. You can start out using bearer tokens which can be setup in 2 minutes. They don't even have to be connected to a database if you don't want to. When you're ready you can switch to more complex solutions like JWT or oAuth. There are many reasons why you should have some sort of an auth solution. Starting from the fact that you can control access to the API and how much someone can access. If something goes wrong, like your API gets spammed, hacked or whatnot you can simply shut down the key that was exposed. You can also use the API key to track how the API was integrated, is someone making too many API calls, is the client behaving erratically. Finally you can even use API calls to gather statistical data about your clients, users and API in general.
My point is - treat your API the same way you would your house. I'm sure you have doors which have keys and you only give those keys to important people. Same should be with your API.
Another thing you should be wary of is sending super sensitive data over the network. We talked about this and the importance of using SSL so that the communication is encrypted. That would be step number one. Step number two is don't return data that might be sensitive and doesn't get used in the apps or the website. I'm talking about user addresses, phone numbers, SSN or any other form of identification. If you don't use it then don't send it. If you are using this please consider making sure that the person who is accessing that API and getting those responses is the actual person whose data you are returning. It sounds simple, I know, but in reality people do all sorts of crazy things. Mostly because they think "oh nothing will happen, it's a small API, nobody knows about it"...Trust me on this one. People will find out and they will take advantage if you let them. So don't.
Before we wrap up I'd like to touch on the UUIDs over IDs debate. I was a long time fan of IDs because they are much shorter and faster but the added security and privacy benefit of UUID is more important in my opinion. UUIDs are much safer. You can still have an ID column in your database that is auto incremented and all but when you are exposing models to the APIs try using UUIDs. Short recommendation but could save you a lot of headache.
Last thing I want to touch upon is general infrastructure security. If you are using AWS or Azure you have an advantage. API gateways give you additional security in terms of firewalls and detection of DDoS attacks. Use them if you can. Anything that will allow you to be able to block a given IP or user is something you should always look for. If you are running on traditional servers with Apache on there here are just two super quick tips on how to save yourselves a few headaches down the line.
First one is simple: keep your software updated. Apache, PHP, Composer packages, NPM packages...everything. Make sure you are always running on the latest and greatest software.
Second, by default Apache sends a response header with every request that literally tells your potential attacker which version of Apache you are running on. The header key is called Server
and the default value could be something like: Apache/2.4.1 (Unix)
. What you wanna do here is quickly hide your Apache version.
Simply open: /etc/apache2/conf-enabled/security.conf
and set the ServerTokens
value to Prod
. After that run sudo systemctl restart apache2 and you've just made your server more secure than it was yesterday. Congrats.
Another quick thing you can do while you have the /etc/apache2/conf-enabled/security.conf
open is turn off ServerSignature
. Just set that to Off
and again you are more secure.
As a general rule you should have quarterly security meetings where you talk about things like how to improve security, how to be better, how to stay safe. You don't wanna be hacked, DDoSed or something similar. Trust me.
5. Be Organized
What better way to stay organized than to version your API ๐ Now I know you've read about this one many times and thought something along the lines of "Oh my API is too small and it's used by one client so I won't use versioning". I've been one of you. I've done that. I've said that. Be smarter and use versions on your API. It's the best decision you can make early on.
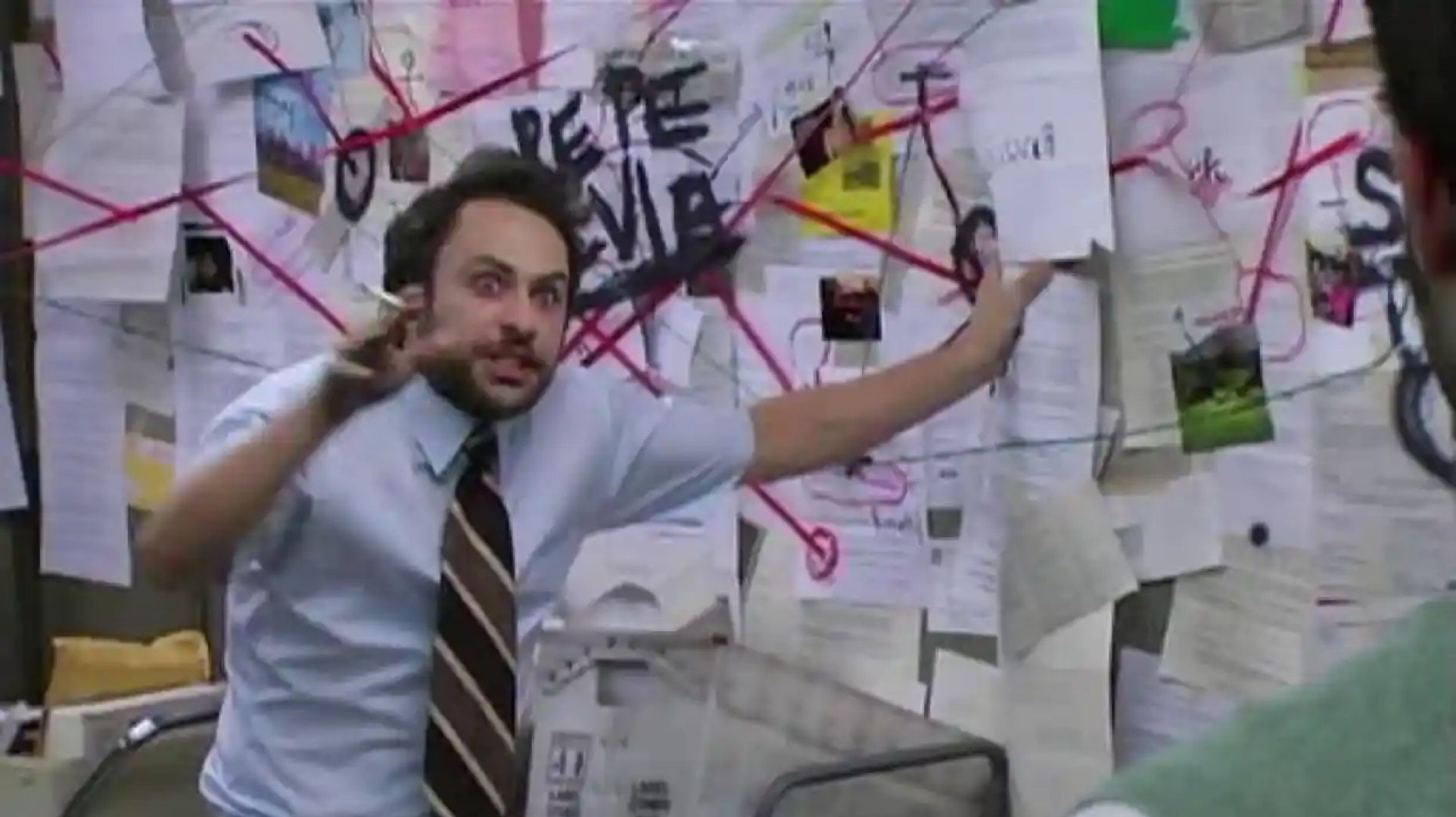
The reason why I was always hesitant towards versioning is because in my mind a jump from v1 to v2 on an API level rarely happens. When I say rarely I mean that in the tech universe - so once a year ๐ Knowing that versioning didn't make sense to me. But boy was I wrong. Yes the big jumps don't happen often but if you have a platform or an app that is being used or developed you'll make smaller updates but more often. I'm talking about weekly or monthly updates to models, data types, structures or processes that are risky to someone who doesn't update the app or something else. To do those, if you don't have versioning enabled, you'll be sweating bullets every time you do a GIT commit. Not only do you have to be sure your code won't break anything or anyone but you need to know how a certain app version will behave. Not fun at all trust me.
Simply choose a versioning scheme that makes the most sense for you and your team. The most common versioning scheme in the software world would be: MAJOR.MINOR.PATCH. In my opinion the PATCH part is a bit too much but you can use that schema if you want. I typically do v1.1 and then all the way up to v1.9. So with a major version you are changing the core parts of the API like authentication, core models, processes etc. With minor and patch versions you're generally adding or removing smaller features, messing with the data structure in some way or something similar.
Another thing that might confuse you is how to actually implement versioning because there are many paths you can take. You could use versioning via: URI paths, request headers, query params or content negotiation. Now each of these has it's pros and cons but I would suggest using URL based versioning. It just makes the most sense and is the most straightforward to understand on so many levels. Everything from making updates, understanding which version is used just from the URL to pointing to the right documentation version. REST APIs are predominantly URI based and I think we should keep up with that tradition and use URI based versioning. Some examples of that include:
- api.domain.com/v1/auth/login
- api.domain.com/v1.2/auth/login
- api.domain.com/v1.4.5/auth/login
- api.domain.com/v2/auth/login
To sum it up. Start using versioning early on and you'll avoid problems later on and be able to scale your API.
6. Be Consistent
You've probably heard the saying: "Consistency is what transforms average into excellence". Well it's true in life as well as API design believe it or not. One of the qualities of a great API is it's consistency. First and foremost I am aiming at resource/model consistency and then other areas like naming, URIs, HTTP codes and similar.
As we know by now APIs boil down to resources. A resource can be anything from a user, article, book, product...anything. Each resource can have multiple properties, objects or arrays. Resources are structured and based on the data you have in the database or some other business logic. Keeping your resource responses consistent is key to your API success. You can't have your endpoints return wildly different resource structures. As much as that sounds tempting or a way to optimize things you should avoid doing that. A mobile developer implementing your API will follow the structure that you provide like a bible. If you send something different on each endpoint time he or she will have a really bad day and nobody wants that. So try to always send the same resource structure. If you don't have data then send it as an empty value or object or array. Let's talk practical and let's say we have an "article" resource and sometimes that article might have comments - sometimes it might not. Sometimes it makes sense to load the comments - sometimes it doesn't. That is fine but still try to have a consistent response in terms of your structure.
When you are getting a single article you wanna load the comments as well like so:
{
"status": true,
"message": "Getting details for article with UUID: 5b8f6db5-7848-490e-95a7-f7146dd2e30c",
"article": {
"title": "Sample title 1",
"description": "Sample description 1",
"image": "https://domain.com/image-1,jpg",
"uuid": "eec33d99-e955-408e-a64a-abec3ae052df",
"comments": [
{
"text": "Great article",
"user": {
"name": "John Doe",
"uuid": "5b8f6db5-7848-490e-95a7-f7146dd2e30c"
}
},
{
"text": "Nice one",
"user": {
"name": "Jane Doe",
"uuid": "2ececb69-d208-46c2-b560-531cb716d25d"
}
}
]
}
}
But when you are loading a list of articles or you've just created an article and there are no comments you should return the following:
{
"status": true,
"message": "Article list was a success",
"articles": [
{
"title": "Sample title 1",
"description": "Sample description 1",
"image": "https://domain.com/image-1,jpg",
"uuid": "eec33d99-e955-408e-a64a-abec3ae052df",
"comments": []
},
{
"title": "Sample title 2",
"description": "Sample description 2",
"image": "https://domain.com/image-2,jpg",
"uuid": "b8ee70a8-1128-4670-9368-83953fdf722b",
"comments": []
}
]
}
So in case the client is expecting to see a comments array they will still get it but it will simply be empty. That way you are not changing your model and removing objects/arrays.
You just saved yourself and others a bunch of time just by being consistent.
7. Be Graceful
If you are building APIs it's a given that things will fail. That's fine. That's expected. You shouldn't feel bad if your API has an error or a problem. What you should feel bad about is if you don't provide the details for it and make sure that your API is smarter than everyone else.
Starting from the top one of the most common things I see developers fail to use is HTTP status codes. In case you didn't know HTTP has status codes for pretty much every scenario imaginable. You just need to know which one to use and send it back to the client. There are over 50 various HTTP response status codes and they all have a unique meaning and should be used in unique scenarios. I won't go through all of them but we will list the most common groups. We've got:
- Informational response codes (start with 1xx)
- Success response codes (start with 2xx)
- Redirection response codes (start with 3xx)
- Client error response codes (start with 4xx)
- Server error response codes (start with 5xx)
So really you have all the status you need. Everything from OK, Unauthorized, Not Found, Internal Server Error to I'm a teapot. Yes, that last one is an actual status. Just proves the guys who made HTTPs were the ultimate jokesters!
In any case each of those statuses has a meaning. That meaning is universally accepted and understood. So a developer from China and a developer from Germany will both understand that if you send a status of 401 (Unauthorized) what you mean to say is that the client did not send the correct authentication details. Since we respond with the status code of 401 (Unauthorized) everybody understands that is a client error and it needs to be fixed on the client not the API. Again I'm giving you just one example but the idea is that you should use the appropriate HTTP status code in appropriate situations. Using them will help your API be universally understandable, consistent and standard. Even though REST isn't a standard this is one of those standard things you should do ๐
Once we have the HTTP status codes working for us we need to provide as many details as we can to the clients when things don't work out. We have to do multiple things to make this happen. First we have to be able to predict how our API might fail, what others might do and not do, who won't play by the rules etc...So the first step is to have strong and strict data validation especially before creating content. After you have the data you have to check if the data is valid! This means checking if the IDs actually exist, if the values are what we expect and can store them in the database...Doing all of this and responding with appropriate status codes will make your API a joy to use. So let's say you have an endpoint which accepts a user_id and gets the user profile. If we apply the strategy of predicting what might happen we'd do the following:
- Check to see if the request has the user_id param - if not respond with 400 Bad Request
- Check to see the given user_id actually exists in the system - if not respond with 404 (Not Found)
- If the user_id returns a result respond with 200 (OK)
We had multiple fail safes as you can see and in all of them we responded with the correct and understandable response code.
Finally once we have our response codes setup and we are predicting how the API might fail we simply need to be as expressive as possible. I know it's hard for us developers to be like that but trust me it's one of the best things you can do. When things go wrong our REST API should have a universal error response model. If we have that then client developers can depend on that to provide users with a more in depth explanation of what went wrong. So let's say a user types in an invalid email on their phone. Somehow that gets sent to the API and the API will of course trigger a validation and error and will respond with 400 (Bad Request). Alongside that the API should send a universal error response model that will allow the client to display any and all messages to the end user. So in this case you might return an error message that says: "Invalid email address entered". The client can read that and show it to the user. And again we need to make sure that you can cover any scenario from validation to server errors. To do that it's best if we come up with a universal error model which could be used in any scenario. I propose you use the following:
{
"title": "Invalid data",
"message": "The data you entered is not valid",
"errors": [
{
"field": "email",
"message": "The email address you provided is not real"
},
{
"field": "phone_number",
"message": "You did not enter a phone number at all"
}
]
}
The JSON structure for errors is simple. We have a title and message which provide a general direction of what happened. A lot of times developers don't choose to display full error details to the end-user. They might use an alert modal on the iPhone to just display our message or title. But we are also sending an errors array which can house specific errors with a specific message. Providing detailed error messages will help you as well as other developers working on the API to understand what exactly went wrong. Even if you are not displaying them to the end user you will still be able to view them once you make a request or if you use a service like Treblle.
So remember, make sure you try to predict as many problems as you can. Provide plenty of details on why things failed even if nobody is using those details and use the universally understood language of HTTP response codes.
8. Be Smart
This one is more philosophical than the other ones but in my opinion is one of the pillars of good REST APIs. If you think about the role of the API in a modern day platform, service or an app we can say that the API is the brain of the whole operation. The reason for that is that every client you might have (iPhone app, Android app, website, IoT device) will talk to the same API. This means our API is the most important part of the whole ecosystem and that our API should be able to handle everything. Gracefully, if I may add.
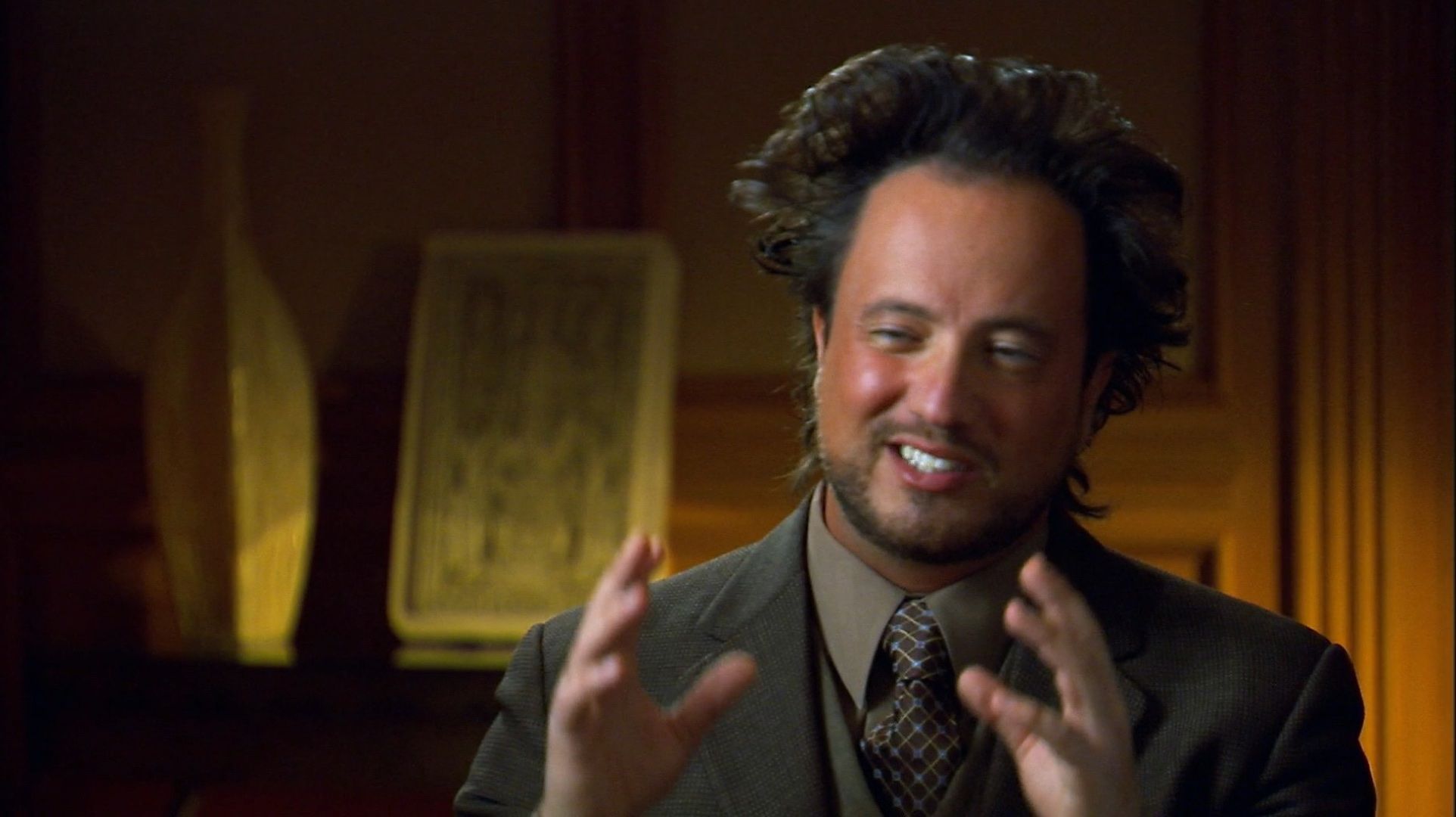
The first thing a smart API should do is protect its most valuable asset - the database. This means it should sanitize, clear out and prevent any bad data from entering the database. The way to do that is by making sure you validate everything that was sent from the app to the client and reject anything that doesn't look good. But when we reject things we should also provide clear reasons to the clients so they know why something happened or in this case didn't happen.
Any good and smart API will handle complex processes on it's own and not depend on clients to help. The simplest example of this would be registering a user in your app. For all the clients that should be one API call where they send the data and they get a user object. But, in the background, the API should handle any and all potential logistics like: register that user on a MailChimp newsletter, store a push token to Firebase, send the user a welcome email and so on...The clients should not be calling multiple API endpoints to those things. If we package everything into one endpoint then you can easily change the flow at any point in time and the clients don't even have to be aware of it. As long as you get the data you need from them the API can always be in full control of the entire business logic and processes.
Make sure that the API is optimized for cross platform solutions. When you are dealing with multiple platforms or devices like iPhones, Android phones, computres, TVs and similar, the API should be able to adjust to them. This means that the API should be flexible enough to handle inputs that might be different from all the clients and still continue to enable the clients to do their job. A good example of this would be resizable images. If you have an API that delivers content which has images you probably don't wanna send a 4000x4000px image to a mobile phone but maybe you would to a TV or a website. A smart API would know which client is accessing that endpoint and would return optimized resources on it's own or even allow the client to request specific sizes. There are many more examples of this but you get the idea.
The less you depend on the client to do things the better. A smart API will always account for the clients imperfections and correct them if possible.
9. Be Lean
What is a good API if not fast and optimized!? Your API should not be the pain point of the entire ecosystem. It's as simple as that. There are many things you can do to make sure you deliver performance and scalability a good API should have. Let's look at some of them.
Being fast and optimized starts on a database level. Whenever I hear someone say their API is slow 9 out of 10 times it has something to do with the database. Either bad database design, complicated queries, slow infrastructure or even the lack of caching. You should always optimize your database structure, queries, indexes and everything else that interacts with your database. Once you have that handled the next thing to focus on is making sure you are delivering cached data whenever possible. Caching database queries will literally reduce your load time by 100% sometimes. So if you have an API endpoint that shows users their profile or something like that I am sure it won't change every 5 minutes. Be smart, cache the data. Your users and the clients calling that API will love it.
Another performance impactor is the sheer amount of data you send down to the clients via the API. Make sure your resources and models are returning only the data the clients need and not the entire shabang. If you are returning a collection of items maybe you don't need the full model details and relationships - everytime. If that will speed things up then go for it but always try to stay consistent with the models returned in the responses. This means that if you are not loading relationships, return an empty array, if you are not loading counts return 0 and so on. Consistency is key when building great REST APIs.
Another pretty simple thing you can do to reduce the response size and increase performance is to enable compression. The most popular one being Gzip compression. On Apache it comes enabled by default with a module called mod_deflate
. When enabled you can usually see a response header of Accept-Encoding
set to something like gzip, compress
. Even though it's enabled by default you still need to "apply" it to your API response. To do that we have to add some rules to our default Apache configuration. So let's actually open the configuration file like so: sudo nano /etc/apache2/conf-available/000-default.conf
and add this thing of beauty: View Gist
That tells Apache to apply Gzip compression on various mime types like application/javascript, application/json and others. Once we save this we simply need to restart Apache by typing in sudo systemctl restart apache2 and we are done. Your API responses are now 50-75% smaller than they were. How easy was that?!
10. Be Considerate
I'll be short with this last piece because weโve gone overboard with the REST (never gets old ๐) but I don't wanna downplay its importance. For us back-end developers the process of developing an API is pretty lonely. Itโs mostly you, a bunch of code and your favorite testing tool. You might think the job is done when you write that last line of code and make a merger into the production branch. But it's not. For many others the journey is just starting.
There are many people that will only start doing their job after you have completed yours. In order for them to even be able to do a good job you need to prepare your API in many ways. You have to make sure itโs working, itโs very well documented and more importantly you have to be ready to provide integration support. No matter how good you write the documentation there will always be questions, doubts and problems during the integration process and beyond.
Put yourself in the shoes of others and try to make their job easier. Build a good API, follow the rules we defined here, write great documentation and be there for everyone else.
Consider using tools that can help you with many of the challanges of building, shipping and running an API. One of those tools is, you guessed it, Treblle. Just by adding Treblle to your API you'll get auto generated documentation with OpenAPI Spec support. Then you get real time monitoring and logging so you can see what others are doing and better help them. Finally you'll even get a quality core of your API based on these same rules. Of course, we've designed it with the entire team in mind. Because we know seeing is believing here is a video on how we can help:
Hopefully I've been able to simplify and explain some of the usual doubts and concerns you might have when building REST APIs. Again, I have to note, REST is not a standard so nobody can tell you you are doing it wrong. But just consider this: daily, as developers, we look for patterns to make our code better, prettier, more efficient so why not do the same with APIs. If we started following some patterns we'd all enjoy a much more healthier and prettier ecosystem.
Finally, I'd like to finish off by quoting a great man who has been like an uncle to all of us - Ben Parker. He once wisely said: "With great power comes great responsibility". That could not be more true when we talk about REST APIs.
PS. If you like these articles say hello on my Twitter or follow me on LinkedIn.