You've probably integrated an AI API into your application, only to discover its limitations when faced with real production workloads. Features listed in documentation often tell only half the story.
Our previous blog post covered several popular AI APIs based on their documented capabilities, but documentation alone doesn't reveal how these APIs perform under pressure.
Now, we will examine these APIs through a more technical lens.
When you select an API for production use, you need concrete data on its performance, security practices, and architectural design. A poorly designed API costs extra development time, introduces security vulnerabilities and creates a technical debt that grows with each integration point.
We tested OpenAI, Hugging Face, AssemblyAI, and Cohere APIs using API Insights to measure what matters: response times, security vulnerabilities, RESTful design adherence, and structural elements that impact integration efficiency.
Each API received scores across four technical categories directly affecting your development experience and application stability.
These technical insights go beyond feature lists to help you make informed architectural decisions based on measurable API quality metrics. You'll find the actual scores for each API alongside detailed explanations of the technical issues we uncovered during testing.
What is API Insights and Why Should You Care?
API Insights is a governance tool by Treblle that evaluates APIs against industry best practices. When you upload an OpenAPI specification (in JSON or YAML format), it generates a score (1-100) and grade (A-F) across four critical dimensions, including AI Readiness—a new feature designed to assess how well an API supports AI/LLM integration.
These scores help you understand the areas where your API meets the standards and where it falls short. The tool runs multiple checks against each category, comparing the results to industry standards.
Example: DEMO API Report by API Insights. This report analyzes the Demo API, which has 33 endpoints.
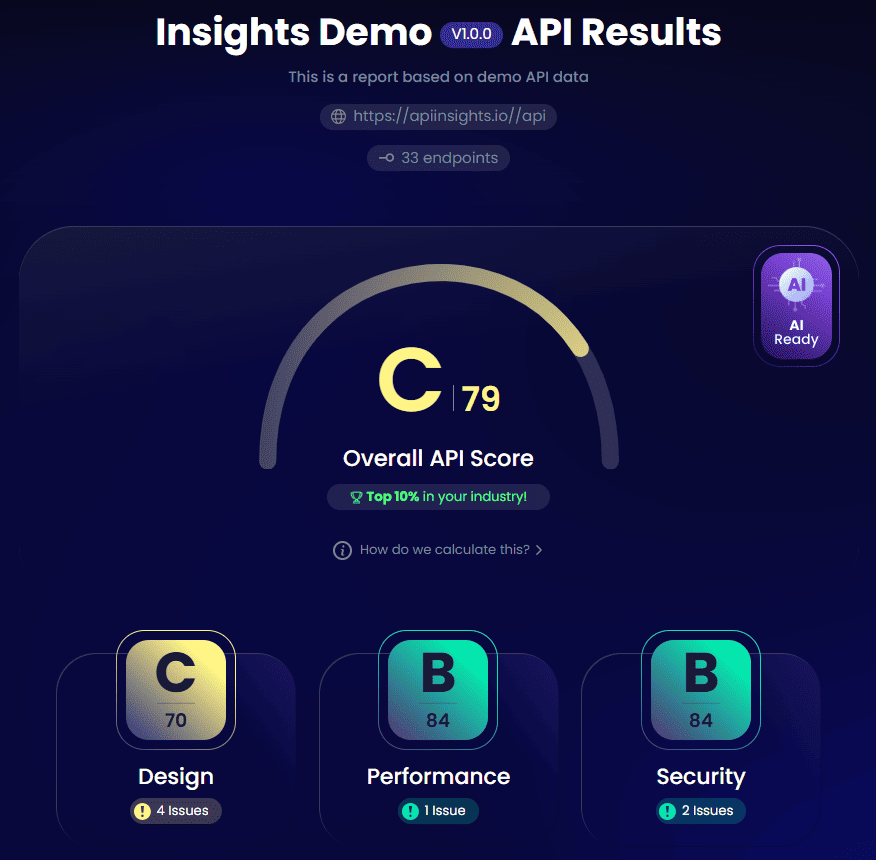
- API Design: Analyzes adherence to RESTful conventions and documentation quality
- API Performance: Measures response times and optimization techniques
- API Security: Identifies vulnerabilities and checks for proper authentication
- AI Readiness: Assesses how well-structured the API is for AI/LLM integration
For developers, this tool answers critical questions:
- Will this API scale under load?
- Is it secure enough for production?
- Can my team integrate it without weeks of trial and error?
We wrote extensively on how API Insights helps developers navigate the API landscape.
How to Use API Insights
- Audit APIs Before Integration: Upload the OpenAPI spec and review the scorecard.
- Enforce Internal Standards: Use the “AI Readiness” checklist to ensure LLM compatibility.
- Monitor Security: Regularly check for vulnerabilities like IDOR risks.
Check the API Insights Documentation to get started. Additionaly, read our guide on how API Insights helps detect OpenAPI issues.
The AI APIs Under the Microscope
1. OpenAI API
The OpenAI API provides access to models like GPT-3 and Codex for text understanding and generation. It powers everything from chatbots to content creation tools and code assistants.
2. Hugging Face Inference API
The Hugging Face Inference API is a gateway to thousands of pre-trained NLP models for tasks like text classification, summarization, and generation. Many research teams and startups use it to deploy state-of-the-art NLP capabilities quickly.
3. AssemblyAI
The AssemblyAI API specializes in speech-to-text conversion, and this API offers transcription services with additional features like sentiment analysis and keyword extraction.
4. Cohere API
The Cohere API focuses on natural language understanding, using models for sophisticated text processing applications such as semantic search and content classification.
We could only test these four AI APIs because they provide public OpenAPI specifications. Many AI APIs don't publish these specs, making governance assessment difficult.
The Test Results
1. OpenAI API (Score: 63/100 - Grade D)
- OpenAPI Specification: Available on GitHub.
- Check API Insights Report.
AI Readiness: 60/100 (D)
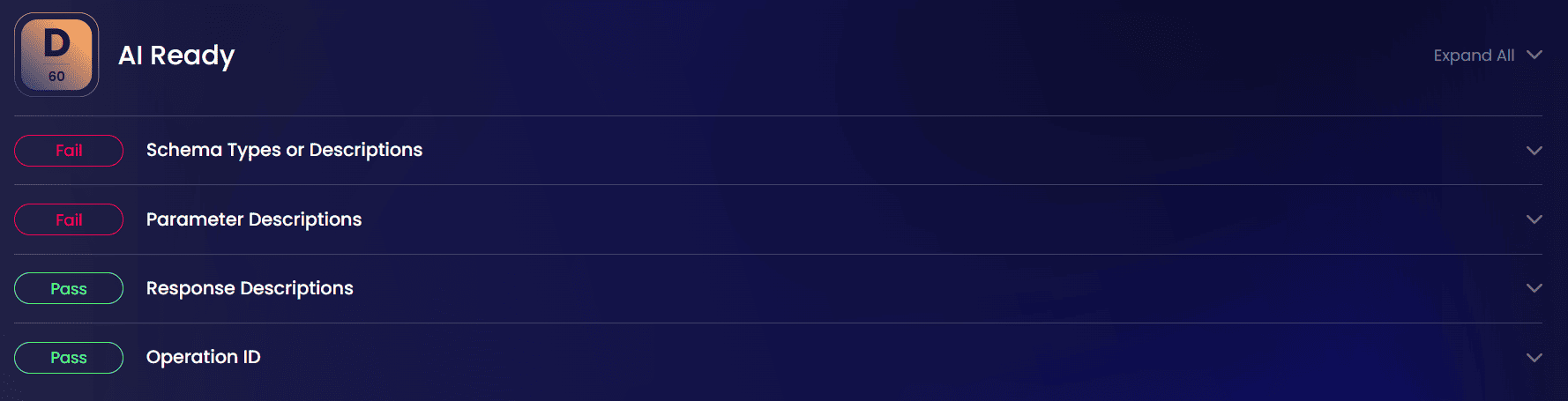
OpenAI's API documentation lacks critical schema and parameter descriptions that would help developers understand the structure of request and response objects. You must spend extra time experimenting with the API to understand all possible parameters and return values.
Design: 60/100 (D)
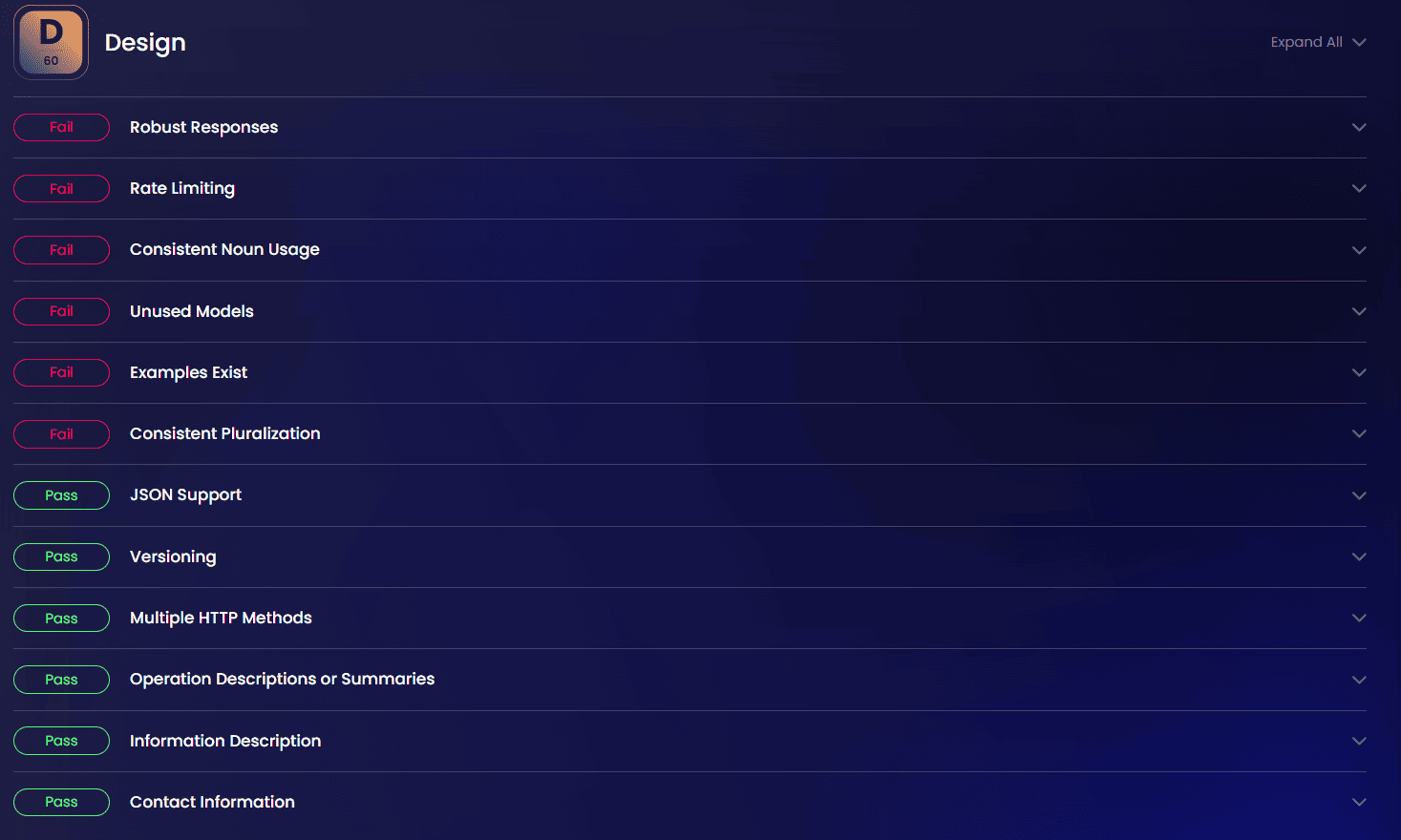
OpenAI's API relies heavily on generic 200 OK responses without properly utilizing HTTP status codes. This forces you to parse error messages from the response body rather than handling errors based on standard HTTP status codes, complicating your error-handling logic.
Performance: 68/100 (D)
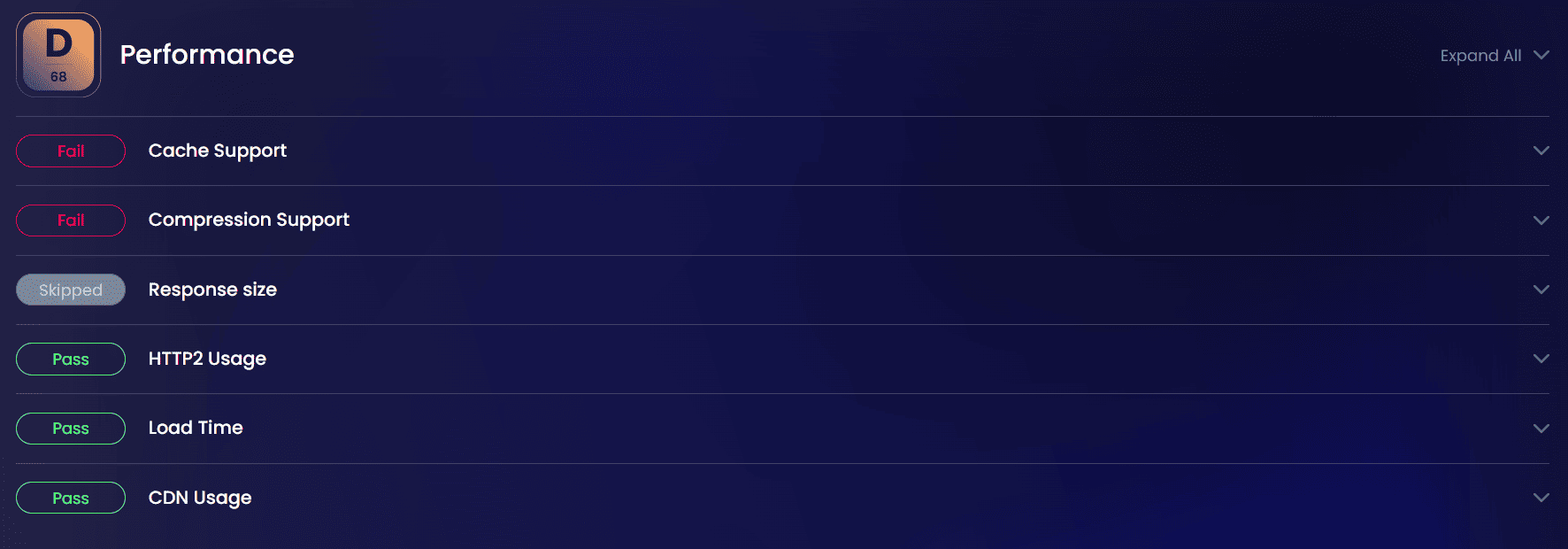
While OpenAI uses a CDN and HTTP/2 for better performance, their lack of cache control headers means your application will repeatedly request resources that could be cached. You must implement your caching layer to avoid unnecessary API calls.
Security: 68/100 (D)
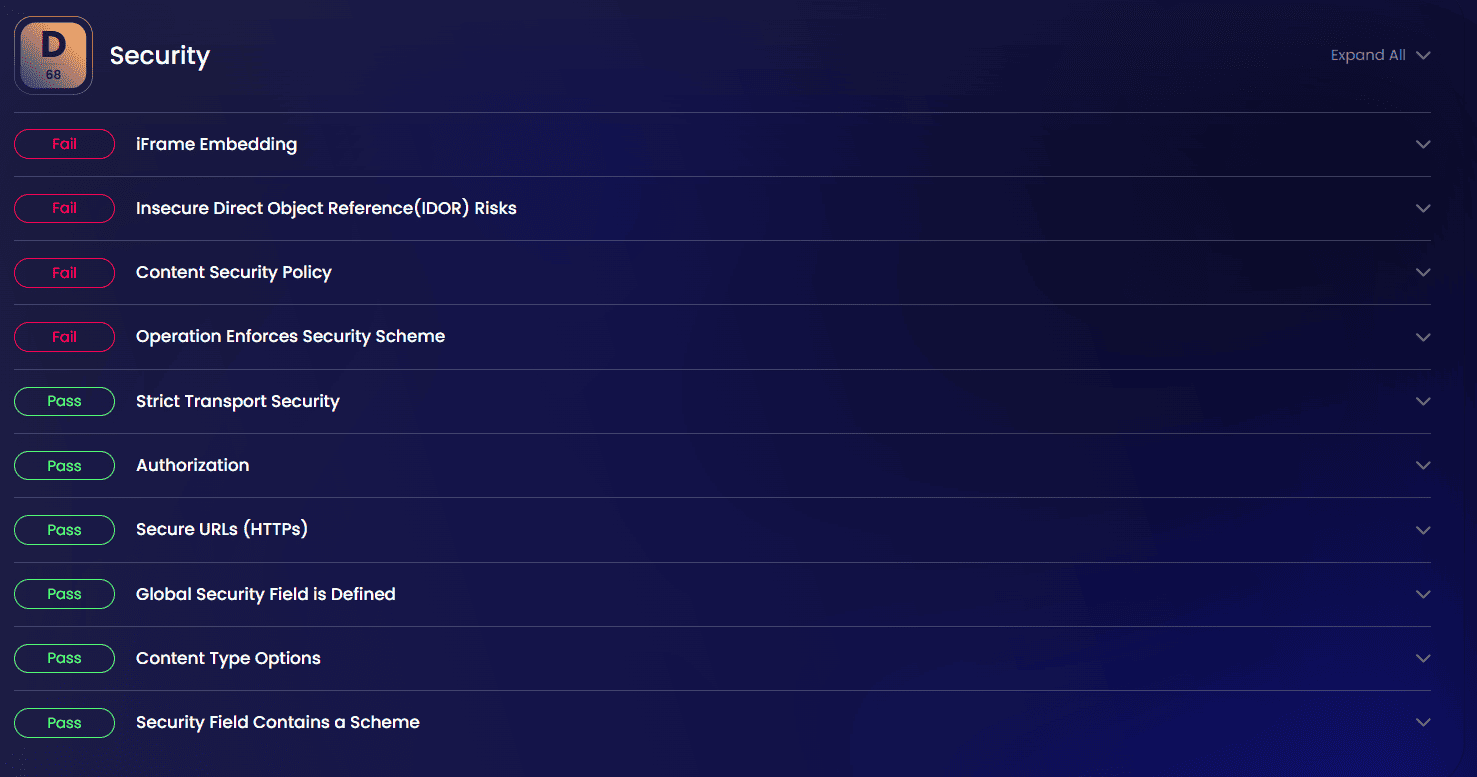
OpenAI's API lacks several essential security headers, such as Content-Security-Policy and X-Frame-Options. You should implement these headers in your application when integrating with OpenAI to prevent potential security vulnerabilities.
2. Hugging Face Inference API (Score: 63/100 - Grade D)
- OpenAPI Specification: API Reference.
- Check API Insights Report.
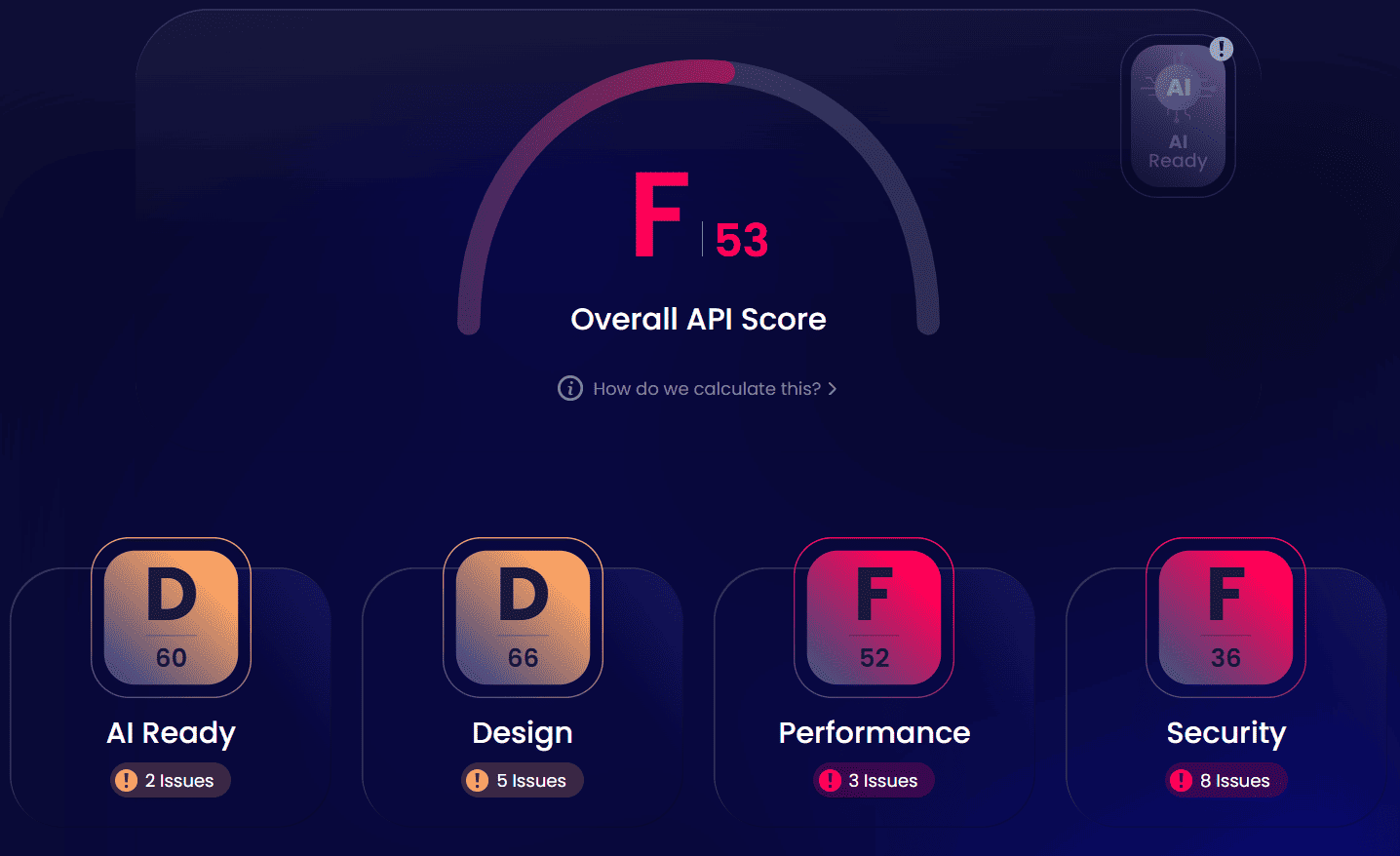
AI Readiness: 60/100 (D)
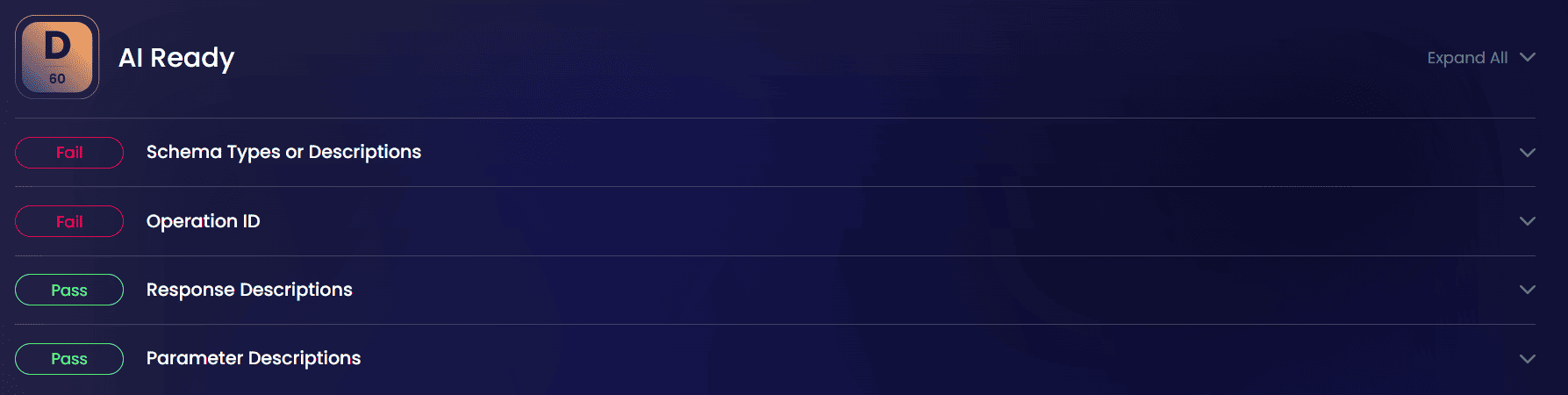
Hugging Face provides decent parameter descriptions but lacks operation IDs, making referencing specific endpoints in your code complex. You must create constants or enums to maintain consistent endpoint references in your application.
Design: 66/100 (D)
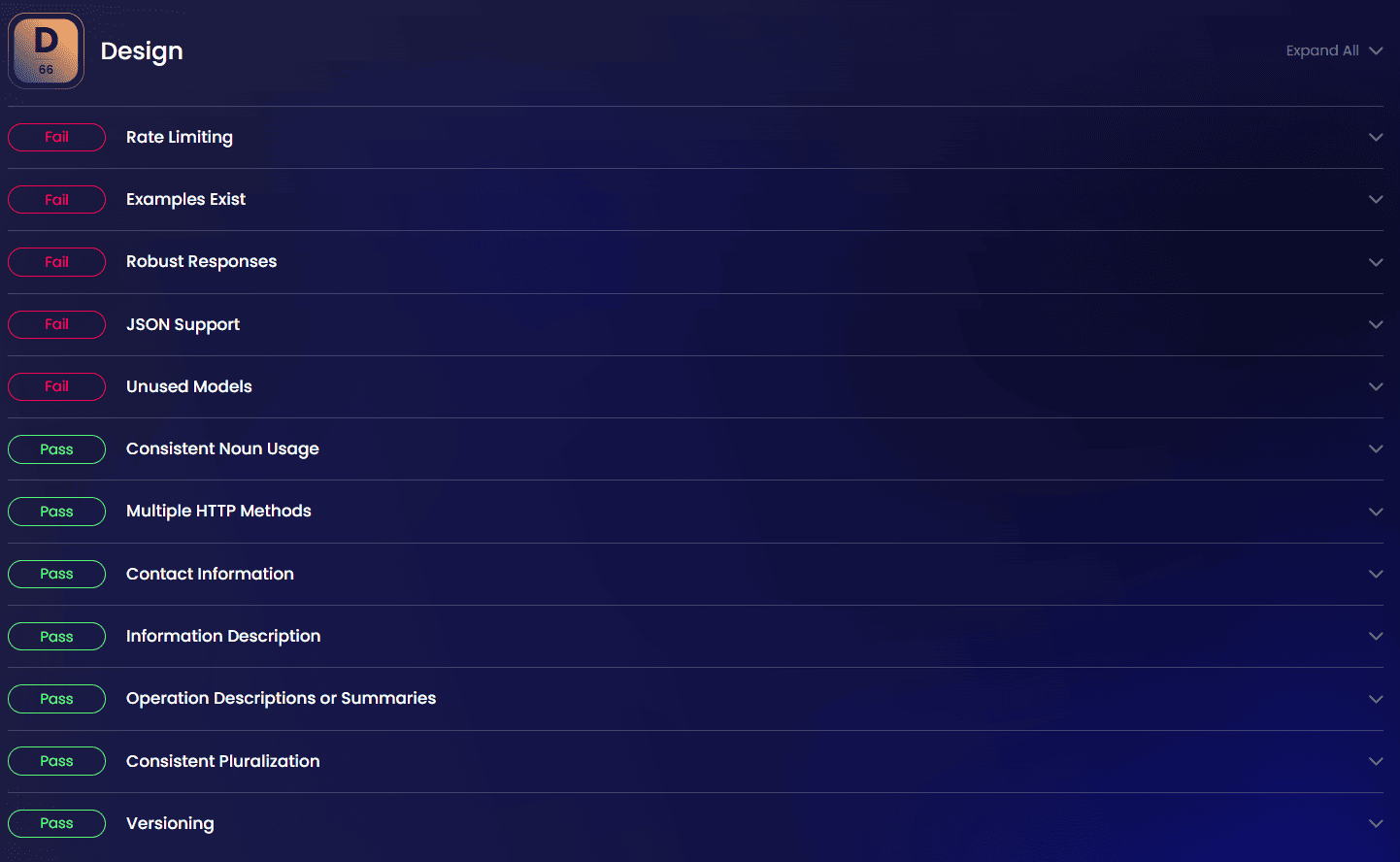
The lack of example responses in Hugging Face's API documentation means you must make test calls to understand the exact response format. Before building production integrations, you should create a test harness to capture and document these responses for your team.
Performance: 52/100 (F)
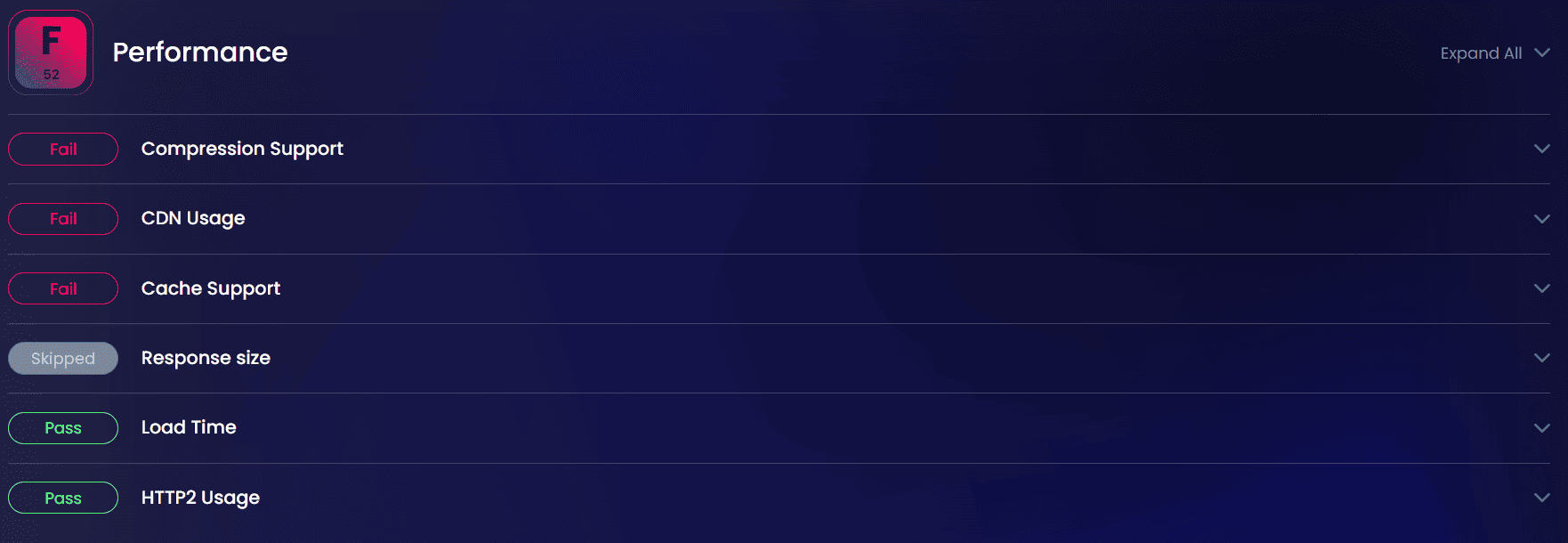
Hugging Face's API performs poorly in terms of optimization. Due to the lack of a CDN and compression support, you will experience slower response times. For production applications, consider implementing request batching to minimize the impact of these performance issues.
Security: 36/100 (F)
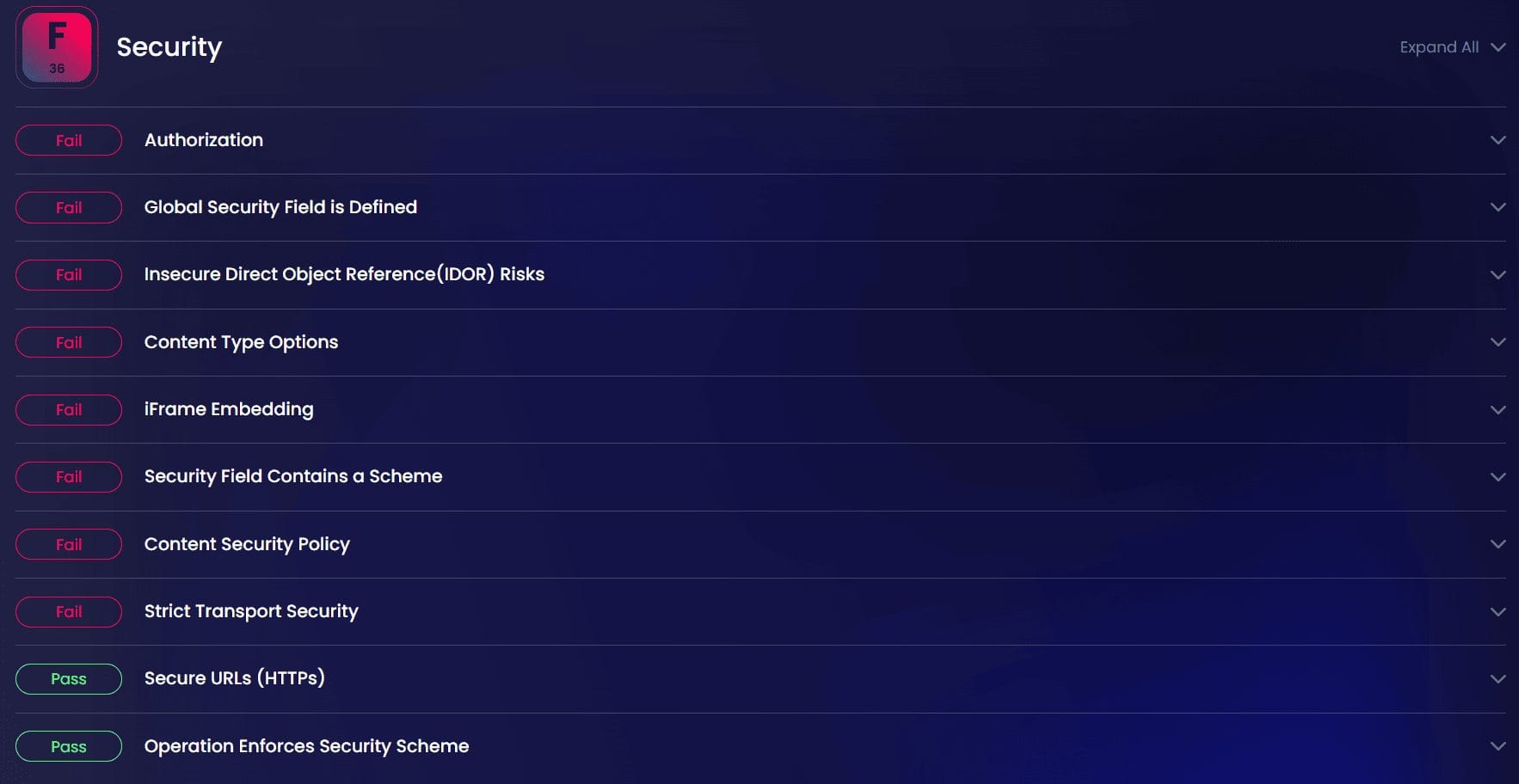
Hugging Face's API received the lowest security score, with multiple critical vulnerabilities detected. To mitigate these risks, you must implement additional security measures in your application, including request validation, proper API key management, and security headers.
3. Cohere API (58/100 - Grade F, 36 endpoints)
- OpenAPI Specification: Available on GitHub.
- Check API Insights Report.
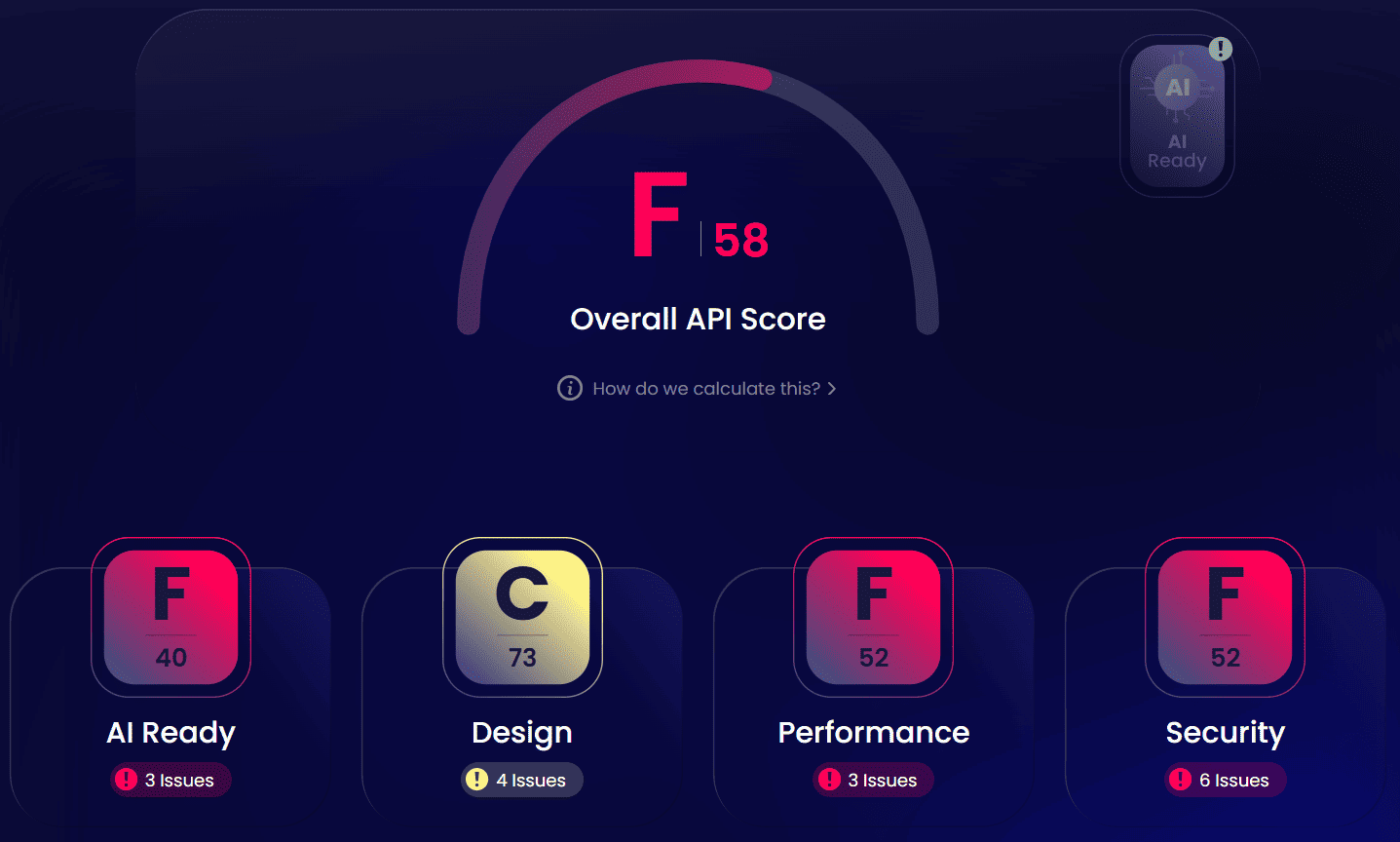
AI Readiness: 40/100 (F)
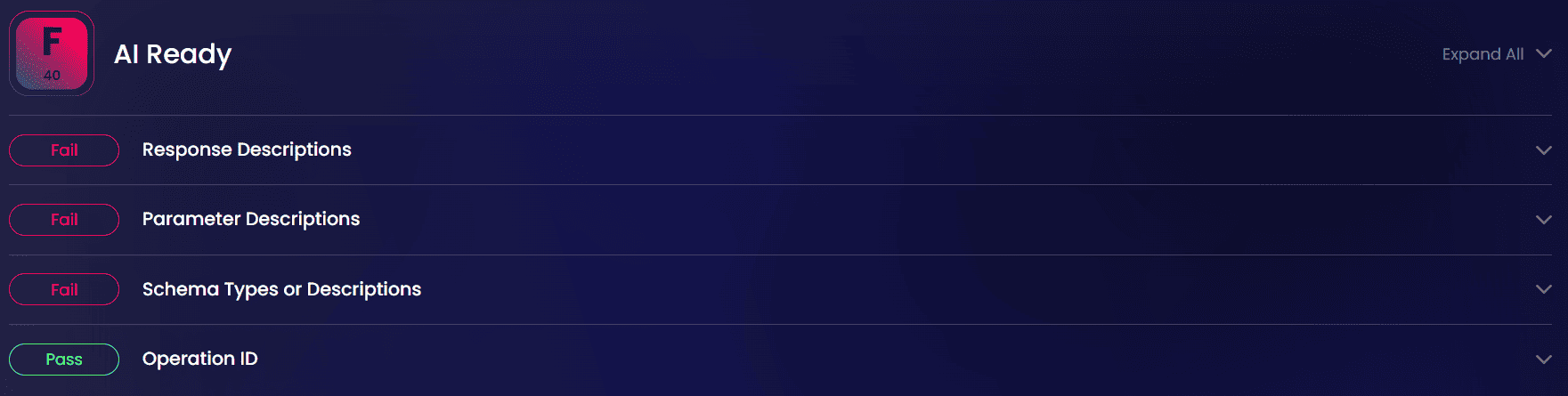
Cohere's API documentation lacks comprehensive parameter and schema descriptions, making it challenging to understand the full capabilities of each endpoint. You must reference their external documentation and conduct exploratory testing to fill these documentation gaps.
Design: 73/100 (C)
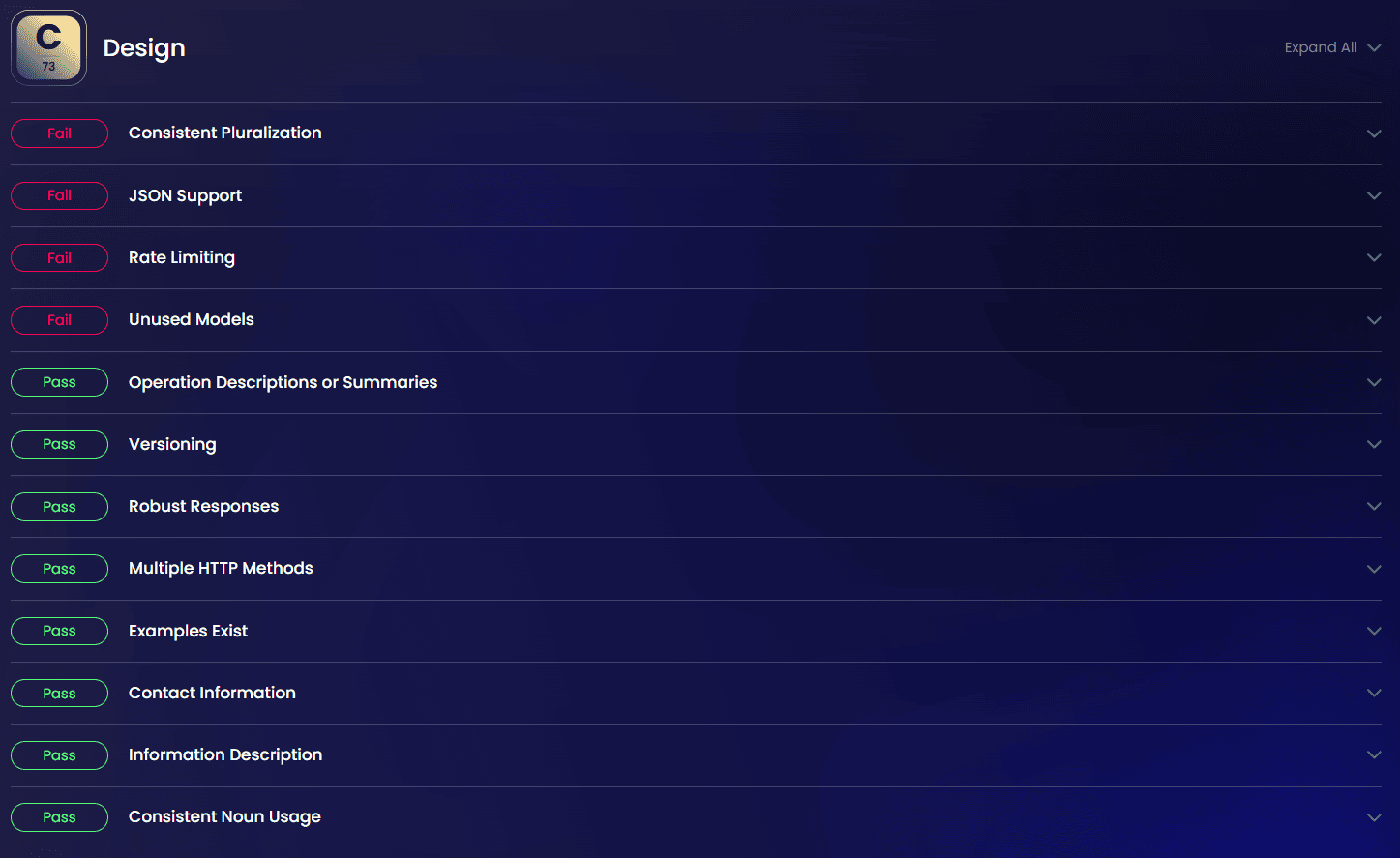
Cohere achieved the best design score among the tested APIs with good examples and consistent naming. However, inconsistent resource pluralization (mixing singular and plural resource names) will require special attention when building URL paths in your application.
Performance: 52/100 (F)
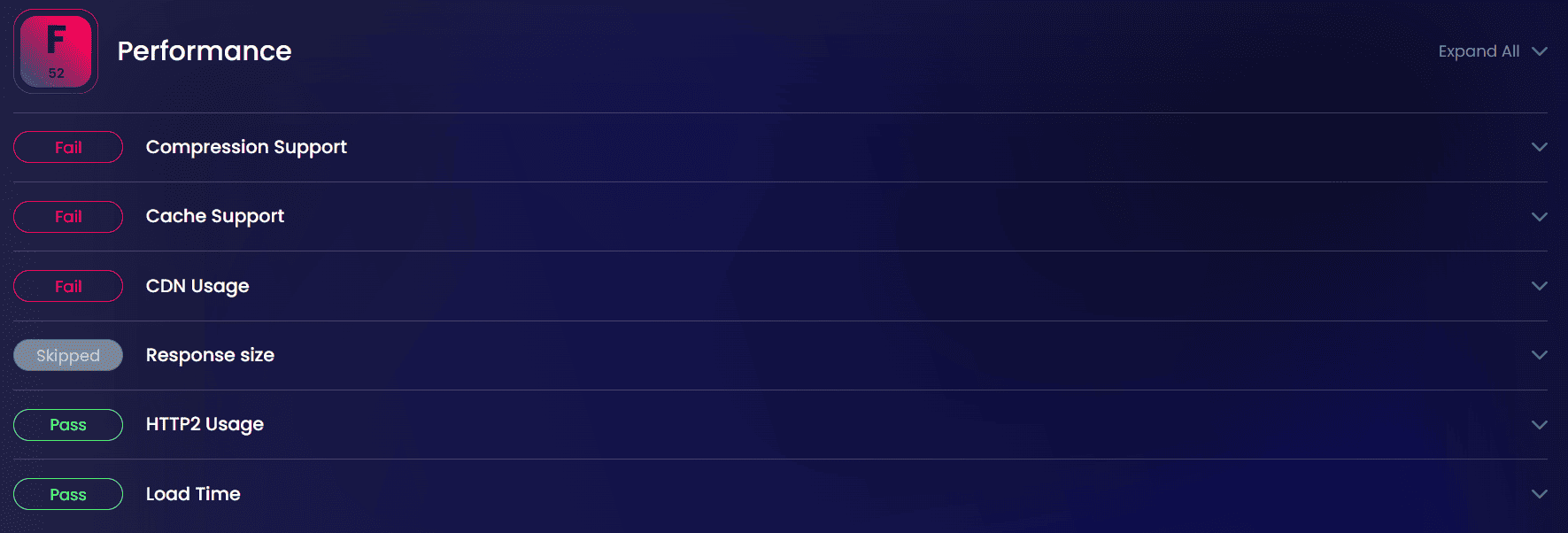
Cohere's API performs adequately for low-volume requests but lacks the infrastructure for high-throughput applications. When building applications with higher traffic demands, you should implement client-side throttling and caching to compensate for these performance limitations.
Security: 52/100 (F)
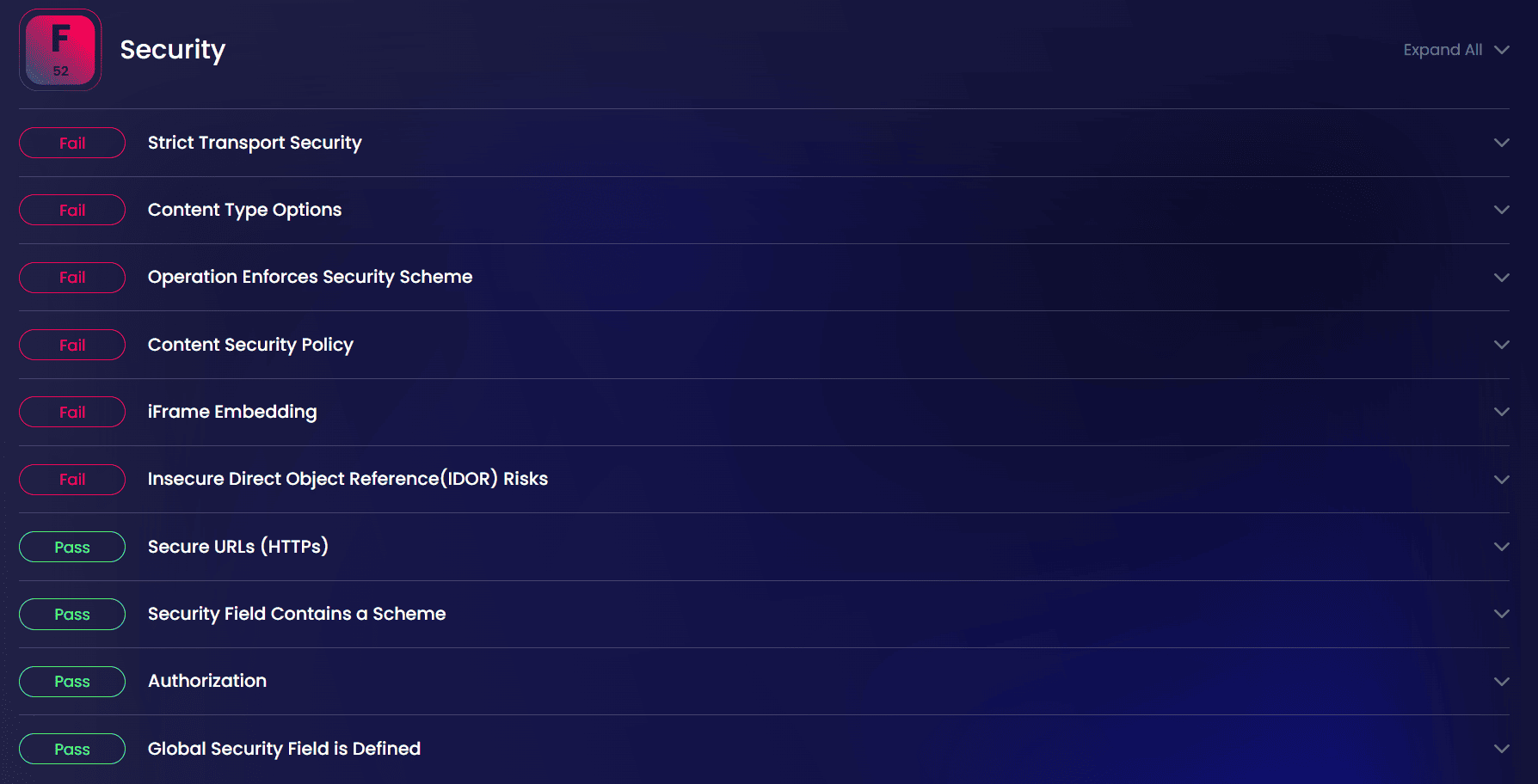
While Cohere implements basic authorization, the IDOR vulnerabilities indicate potential security issues with resource ID validation. You must implement additional validation on resource IDs received from the API to prevent possible security exploits.
4. AssemblyAI API (61/100 - Grade D)
- OpenAPI Specification: Available on GitHub.
- Check API Insights Report.
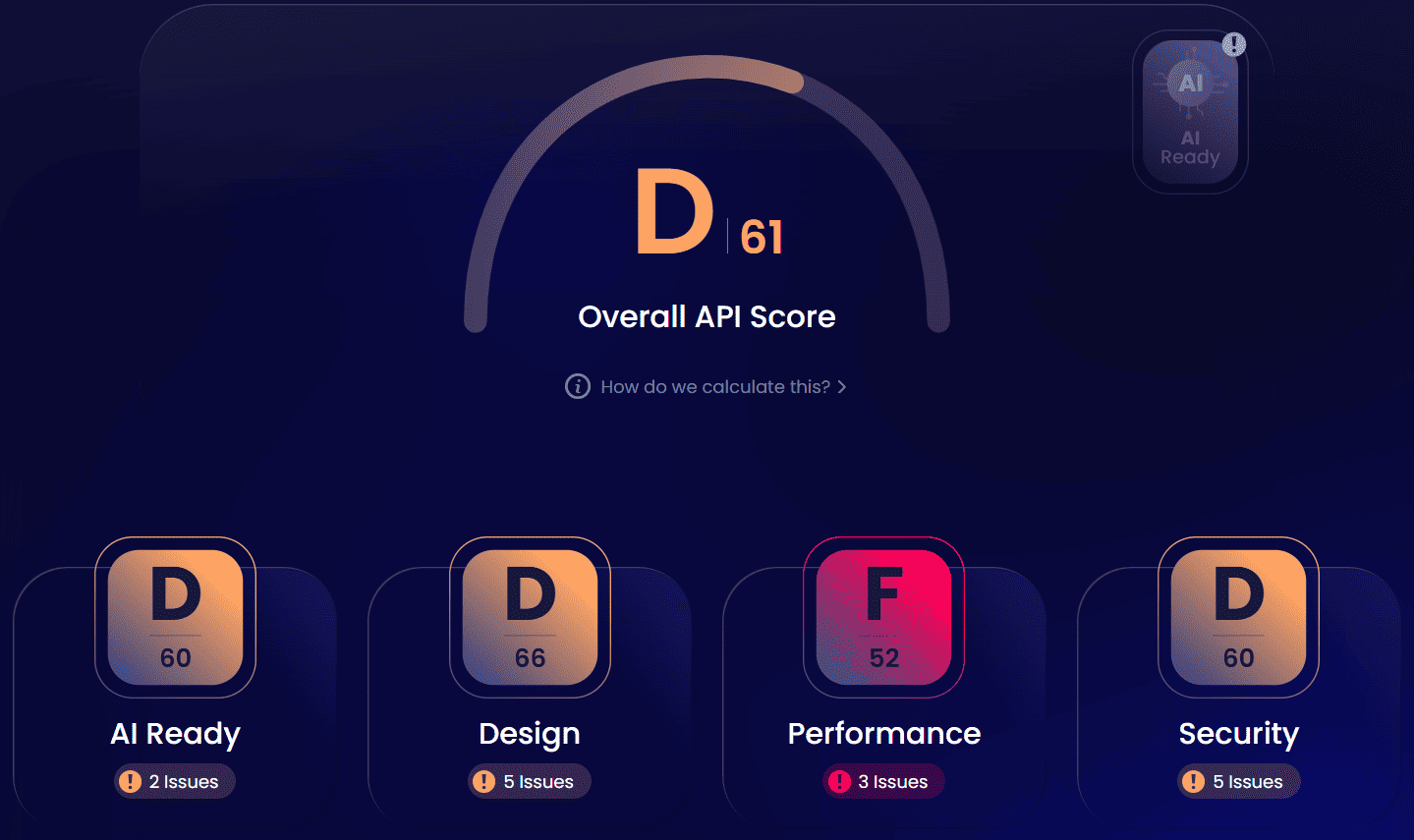
AI Readiness: 60/100 (D)
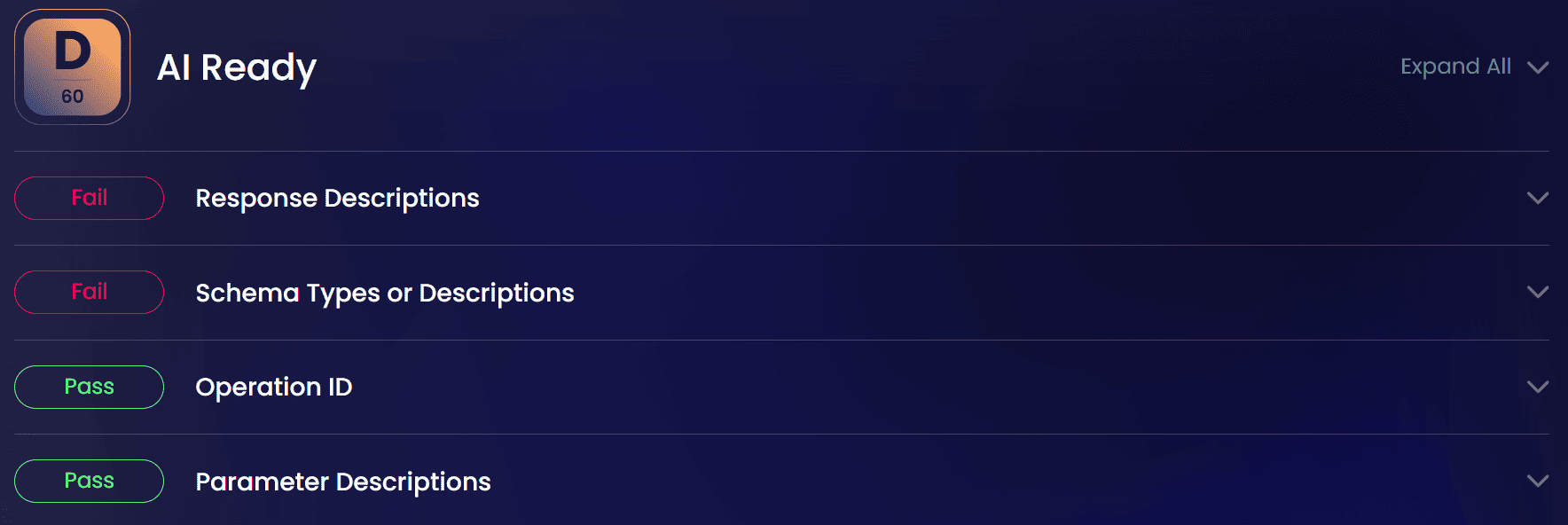
AssemblyAI provides good parameter descriptions but lacks response descriptions. As the structure isn't well documented, you will need to handle responses defensively, checking for the existence of fields before using them.
Design: 66/100 (D)
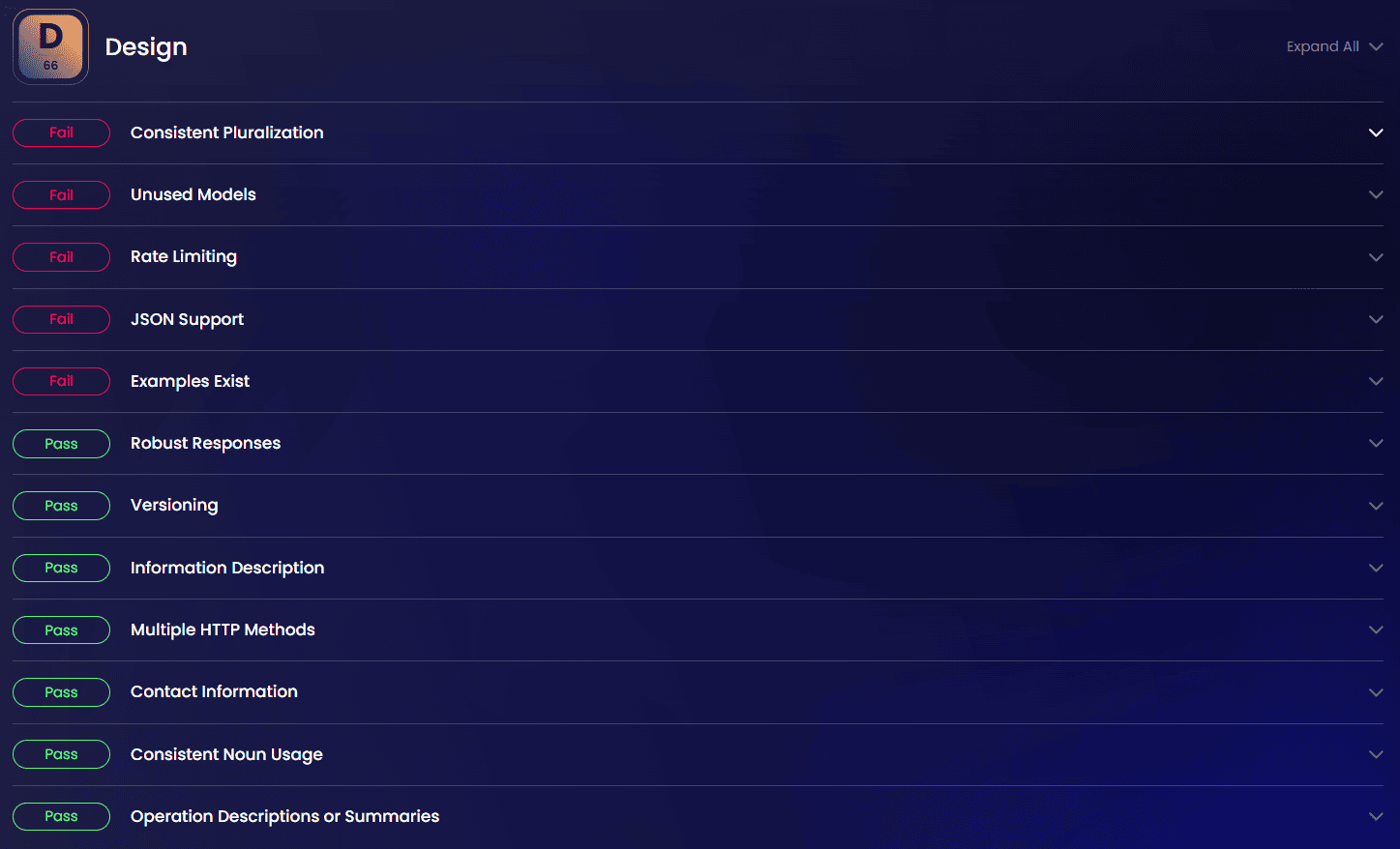
AssemblyAI effectively uses HTTP status codes, which makes error handling more straightforward. However, lacking examples means you must experiment with each endpoint to understand the expected request format and response structure.
Performance: 52/100 (F)
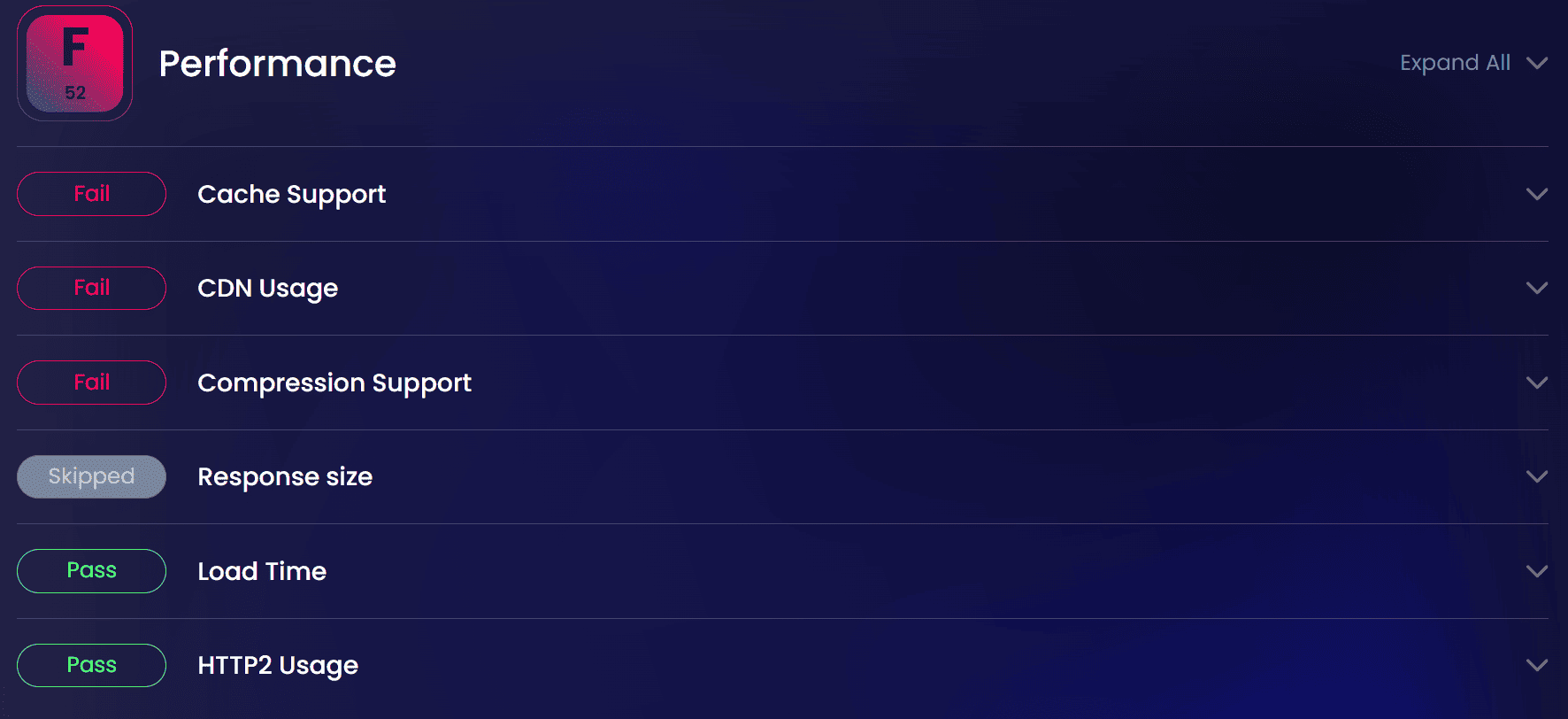
For audio-heavy applications using AssemblyAI, the lack of compression support is particularly problematic. You should implement client-side compression of audio files before transmission to improve upload performance and reduce bandwidth costs.
Security: 60/100 (D)
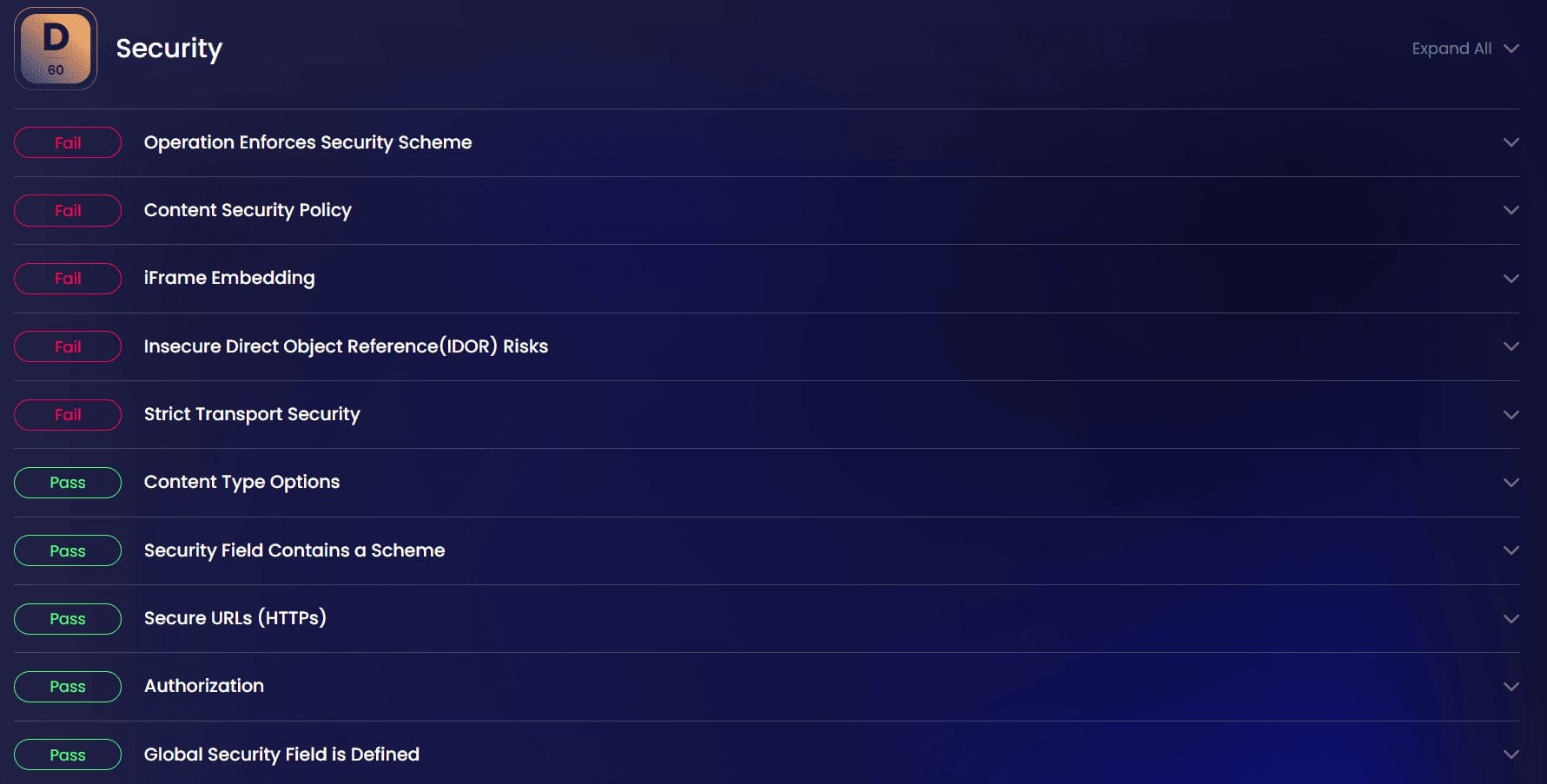
AssemblyAI implements proper authorization mechanisms but lacks necessary security headers. When integrating with this API, add security headers to your application's responses and implement additional validation for resource IDs.
What This Means for Developers
These findings highlight important considerations when choosing and implementing AI APIs:
- Documentation Gaps Require Additional Testing: Even top-tier AI APIs have incomplete OpenAPI specifications. When integrating, allocate extra time for discovering undocumented behavior through systematic testing. Check out this guide on API documentation best practices and tools.
- Implement Additional Security Layers: Don't rely solely on the API's built-in security. When building applications that consume these APIs, add your validation, rate limiting, and security headers.
- Build Performance Optimizations: Since most APIs lack proper caching and compression, implement your caching layer and response compression to improve application performance.
- Test Thoroughly Before Committing: Governance scores indicate potential issues, but nothing replaces thorough testing with real-world data and traffic patterns in your specific use case.
Conclusion
The results show that even the most popular AI APIs have significant room for improvement in governance, particularly in security and AI readiness. Before selecting an API for your project, consider running your own API Insights analysis and testing.
For developers building their APIs, these findings offer valuable lessons:
- Be properly documented with schema descriptions and examples for easy integration.
- Maintain strong security practices, including authentication, validation, and security headers.
- Optimize performance with correct caching, compression, and efficient request handling.
- Follow RESTful design principles to ensure consistency across endpoints.
- Implement API Governance best practices.
Tools like API Insights let you:
- Compare APIs objectively: Use scores to prioritize options.
- Fix Issues Early: Address design flaws before they reach production.
Choosing the right API isn’t just about features—it’s about long-term reliability, security, and ease of integration. With API Insights, you gain the clarity needed to build with confidence and avoid costly surprises down the road.